EmployeeDetail_Dataset.WriteXml("c:\temp.xml")
now xml reflecting the dataset will be in c:\temp.xml
more...
Wednesday
dot.net application or dot.net dll location VB.NET/C#
here's few ways to get application/dot net dll location :
System.AppDomain.CurrentDomain.BaseDirectory
System.AppDomain.CurrentDomain.SetupInformation.ApplicationBase
for ASP.NET u can use following :
Server.MapPath(".")
more...
System.AppDomain.CurrentDomain.BaseDirectory
System.AppDomain.CurrentDomain.SetupInformation.ApplicationBase
for ASP.NET u can use following :
Server.MapPath(".")
more...
Javascript log/debug window for client-site logging
1.Add this code to page or include
use log('my message') function in javascript to show data in window during javascript execution.
more...
var okno;
okno=window.open("", "Debug", "left=0,top=0,width=300,height=700,scrollbars=yes," +"status=yes,resizable=yes");
function log(msg){
okno.document.write("<br>"+msg);
}
use log('my message') function in javascript to show data in window during javascript execution.
more...
Tuesday
Regular Expressions - Strong Password Validation
string r =
@"(?x)" + // Ignore spaces within regex expression, for readability
@"^" + // Anchor at start of string
@"(?=.* ( \d | \p{P} | \p{S} ))" + // String must contain a digit or punctuation char or symbol
@".{6,}"; // String must be at least 6 characters in length
Console.WriteLine (Regex.IsMatch ("abc12", r));
Console.WriteLine (Regex.IsMatch ("abcdef", r));
Console.WriteLine (Regex.IsMatch ("ab88yz", r));
more...
Grouping with LINQ to SQL filtered
// The following groups purchases by year, then returns only those groups where
// the average purchase across the year was greater than $1000:
from p in Purchases
group p.Price by p.Date.Year into salesByYear
where salesByYear.Average (x => x) > 1000
select new
{
Year = salesByYear.Key,
TotalSales = salesByYear.Count(),
AvgSale = salesByYear.Average(),
TotalValue = salesByYear.Sum()
}
more...
LINQ Join vs SelectMany:what is faster?
Customer[] customers = Customers.ToArray();
Purchase[] purchases = Purchases.ToArray();
var slowQuery =
from c in customers
from p in purchases where c.ID == p.CustomerID
select c.Name + " bought a " + p.Description;
var fastQuery =
from c in customers
join p in purchases on c.ID equals p.CustomerID
select c.Name + " bought a " + p.Description;
slowQuery.Dump ("Slow local query with SelectMany");
fastQuery.Dump ("Fast local query with Join");
more...
Monday
convert sources into html /free
Generate XML with LINQ (C#)
var customers =
new XElement ("customers",
from c in Customers
select
new XElement ("customer", new XAttribute ("id", c.ID),
new XElement ("name", c.Name),
new XElement ("buys", c.Purchases.Count)
)
);
customers.Dump();
more...
command line xml parser ( free, open source)
located @ http://xmlstar.sourceforge.net/
more...
XMLStarlet Toolkit: Command line utilities for XML
Usage: xml [] [ ]
whereis one of:
ed (or edit) - Edit/Update XML document(s)
sel (or select) - Select data or query XML document(s) (XPATH, etc)
tr (or transform) - Transform XML document(s) using XSLT
val (or validate) - Validate XML document(s) (well-formed/DTD/XSD/RelaxNG)
fo (or format) - Format XML document(s)
el (or elements) - Display element structure of XML document
c14n (or canonic) - XML canonicalization
ls (or list) - List directory as XML
esc (or escape) - Escape special XML characters
unesc (or unescape) - Unescape special XML characters
pyx (or xmln) - Convert XML into PYX format (based on ESIS - ISO 8879)
p2x (or depyx) - Convert PYX into XMLare:
--version - show version
--help - show help
Wherever file name mentioned in command help it is assumed
that URL can be used instead as well.
Type: xml--help for command help
XMLStarlet is a command line toolkit to query/edit/check/transform
XML documents (for more information see http://xmlstar.sourceforge.net/)
more...
Sunday
how to change header in blogger
U can create script like that :
more...
<script type="text/javascript">
var container = document.getElementById("header-inner");
container.innerHTML ="http://sourcefield.blogspot.com";
</script>
more...
Monday
Service has zero application (non-infrastructure) endpoints FIX
During installation of the webservice I discovered inresting error:
This is more IIS issue, webserver has default directory that was deleted and currently not exists.
That caused the problem,so after changing default directory all started working.
more...
WebHost failed to process a request.
Sender Information: System.ServiceModel.Activation.HostedHttpRequestAsyncResult/25773083
Exception: System.ServiceModel.ServiceActivationException: The service '/Business/Service.svc' cannot be activated due to an exception during compilation.
The exception message is: Service 'Business.WebService.Service' has zero application (non-infrastructure) endpoints. This might be because no configuration file was found for your application, or because no service element matching the service name could be found in the configuration file, or because no endpoints were defined in the service element..
System.InvalidOperationException: Service 'Business.WebService.Service' has zero application (non-infrastructure) endpoints. This might be because no configuration file was found for your application, or because no service element matching the service name could be found in the configuration file, or because no endpoints were defined in the service element.
at System.ServiceModel.Description.DispatcherBuilder.EnsureThereAreNonMexEndpoints(ServiceDescription description)
at System.ServiceModel.Description.DispatcherBuilder.InitializeServiceHost(ServiceDescription description, ServiceHostBase serviceHost)
at System.ServiceModel.ServiceHostBase.InitializeRuntime()
at System.ServiceModel.ServiceHostBase.OnBeginOpen()
at System.ServiceModel.ServiceHostBase.OnOpen(TimeSpan timeout)
at System.ServiceModel.Channels.CommunicationObject.Open(TimeSpan timeout)
at System.ServiceModel.ServiceHostingEnvironment.HostingManager.ActivateService(String normalizedVirtualPath)
at System.ServiceModel.ServiceHostingEnvironment.HostingManager.EnsureServiceAvailable(String normalizedVirtualPath)
--- End of inner exception stack trace ---
at System.ServiceModel.AsyncResult.End[TAsyncResult](IAsyncResult result)
at System.ServiceModel.Activation.HostedHttpRequestAsyncResult.End(IAsyncResult result)
Process Name: w3wp
Process ID: 1680
This is more IIS issue, webserver has default directory that was deleted and currently not exists.
That caused the problem,so after changing default directory all started working.
more...
Wednesday
vb6 or asp xsl transform (transformNode)
Private Function Transform(xml_string, xsl_file) As String
Dim xsl, XML
Set xsl = CreateObject("Microsoft.XMLDOM")
Set XML = CreateObject("Microsoft.XMLDOM")
xsl.Load (xsl_file)
XML.LoadXml (xml_string)
Transform = XML.transformNode(xsl)
End Function
more...
Friday
LINQ:check if IEnumerable is empty (CSharp/C#)
public static void NonEmpty<T>(IEnumerable<T> source,string m){
if (source == null) Throw(m);
if (!source.Any()) Throw(m);
}
more...
Local sequence cannot be used in LINQ to SQL implementation of query operators except the Contains() operator
System.NotSupportedException: Local sequence cannot be used in LINQ to SQL implementation of query operators except the Contains() operator
Method has no supported translation to SQL
The cause of these errors may be mismatch b/w server and client side properties.
Or b/w properties those have SQL presentation and that hasn't:
private IQueryable<Invoice> AttachInvoices(LinqDataObjectsDataContext dc,string sInvoices) {
List<InvArg> ral = new List<InvArg>();
ral = (List<InvArg>)CXMLConv.Load(sInvoices, ral.GetType(), "ArrayOfInvArg") ;
IEnumerable<InvArg> ra = ral;
var inv = from i in dc.Invoices
join r in ra on i.InvoiceID equals r.InvoiceID
select i;
return inv;
}
must be modified to :
private List<Invoice> AttachInvoices(LinqDataObjectsDataContext dc,string sInvoices) {
List<InvArg> ral = new List<InvArg>();
ral = (List<InvArg>)CXMLConv.Load(sInvoices, ral.GetType(), "ArrayOfInvArg");
long[] s=ral.Select(p => p.InvoiceID).ToArray();
var inv = from i in dc.Invoices
where s.Contains(i.InvoiceID)
select i;
return inv.ToList();
}
more...
Thursday
display html on vb6 form
1.add Microsoft Internet controls to link reference.
2.Place control on form, I used 'Browser' as control name.
use this function to display :
more...
2.Place control on form, I used 'Browser' as control name.
use this function to display :
Private Sub ShowHtml(s As String)
Browser.Navigate ("about:blank")
' Dim doc As IHTMLDocument2
Set boxDoc = Browser.Document
boxDoc.Write (s)
boxDoc.Close
End Sub
more...
play sound in console or bat file
to play sound in console or bat file use this line
sndrec32.exe /play /embedding c:\i386\Blip.wav
more...
sndrec32.exe /play /embedding c:\i386\Blip.wav
more...
Wednesday
run regsvr32 command for all files in folder
you can create simple bat file with one line
--regdir--
for %%f in (%1\*.ocx) do (regsvr32 /s "%%f")
--end of regdir--
then run >: regdir c:\mylibs
that might be useful if you want to run command for all files
more...
--regdir--
for %%f in (%1\*.ocx) do (regsvr32 /s "%%f")
--end of regdir--
then run >: regdir c:\mylibs
that might be useful if you want to run command for all files
more...
Tuesday
allow opening chm file from network drives
add this key to registry, or copy-paste it into reg file execute:
---Begin Reg FILE--
Windows Registry Editor Version 5.00
[HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\HTMLHelp\1.x\ItssRestrictions]
"MaxAllowedZone"=dword:00000001
---End Reg FILE--
more...
---Begin Reg FILE--
Windows Registry Editor Version 5.00
[HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\HTMLHelp\1.x\ItssRestrictions]
"MaxAllowedZone"=dword:00000001
---End Reg FILE--
more...
Monday
Thursday
content length quota (8192) has been exceeded fix
to fix this error in Acessing WCF service:
do folowing :
1.Open web.config file and add these content under system.serviceModel:
2.Find this line and add bindingConfiguration :
more...
The formatter threw an exception while trying to deserialize the message:
Error in deserializing body of request message for operation 'FreezeInvoices'.
The maximum string content length quota (8192) has been exceeded while reading XML data.
This quota may be increased by changing the MaxStringContentLength property on the XmlDictionaryReaderQuotas object used when creating the XML reader. Line 1,
do folowing :
1.Open web.config file and add these content under system.serviceModel:
<system.serviceModel>
<bindings>
<basicHttpBinding>
<binding name="Binding1" closeTimeout="00:11:00" openTimeout="00:11:00" receiveTimeout="00:10:00" sendTimeout="00:10:00" allowCookies="false" hostNameComparisonMode="StrongWildcard" maxBufferSize="9965536" maxBufferPoolSize="9524288" maxReceivedMessageSize="9965536" messageEncoding="Text" textEncoding="utf-8" transferMode="Buffered" >
<readerQuotas maxDepth="2147483647" maxStringContentLength="2147483647" maxArrayLength="2147483647" maxBytesPerRead="2147483647" maxNameTableCharCount="2147483647" />
<security mode="None" />
</binding>
</basicHttpBinding>
</bindings>
2.Find this line and add bindingConfiguration :
<endpoint address="" binding="basicHttpBinding" contract="Business.WebService.IService" bindingConfiguration="Binding1">
more...
Wednesday
python setdefault in indexing text file
indx={}
with open(filename) as f:
for n,line in enumerate(f):
for word in line.split():
indx.setdefault(word,[]).append(n)
#emit in alphabetical order
for word in sorted(indx):
print "%s:" % word
for n in indx[word]: print n,
more...
python property & dunder special methods
#property sample
class blah(object)
def getter(self):
return ...
def setter(self,value):
....
name=property(getter,setter)
inst=blah()
print inst.name #calls inst.getter()
inst.name=23 #like call inst.setter(23)
python special methods (dobleundescore aka dunder):
__new__ __init__ __del__ # ctor,init, finalize
__repr__ __str__ __int__ # convert
__lt__ __gt__ __eq__ # compare
__add__ __sub__ __mul__ # arithmetic
__call__ __hash__ __nonzero__ # ()
__getattr__ __setattr__ __delattr__ #
__getitem__ __setitem__ __delitem__ #
__len__ __iter__ __contains__ #
__get__ __set__ __enter__ __exit__
here's sample of using special methods
class fib(object):
def __init__(self): self.i=self.j=1
def __iter__(self): return self
def next(self):
r,self.i=self.i,self.j
self.j+=r
return r
for r in fib():
print r,
if r > 100: break
1 1 2 3 5 8 13 21 34 55 89 144
more...
python functions generators closures decorators
Types of arguments:
#default values:
def sub1( a,b='default',i=0)named arguments
def info(spacing=15, object=odbchelper):tuple (args,params)
def sumsq2(*a): return sum(x*x for x in a)dictionary
def sumsq2(**a):generators in python - used yield insted of return
def fibonacci()
i=j=1
while True:
r,i,j = i,j,i+j
yield r
for rabbits in fibonacci():
print rabbits
if rabbit > 100 : break
>>
1 1 2 3 5 8 13 21 34 55 89 144Closures
def makeAdder(addend):
def adder(augend):
return audend + addend
return adder
a23=MakeAdder(23)
a42=MakeAdder(42)
print a23(100) , a42(100) , a23(a42(100))
>> 123 142 165decorators
@<decor>
def <name>
#is like
def (name)
name = <decor>(<name>)
#or another example
@dec2
@dec1
def func(arg1, arg2, ...):
pass
#This is equivalent to:
def func(arg1, arg2, ...):
pass
func = dec2(dec1(func))and singleton example
def singleton(cls):
instances = {}
def getinstance():
if cls not in instances:
instances[cls] = cls()
return instances[cls]
return getinstance
@singleton
class MyClass:
...
more...
XElement.Parse using and create xml with linq:
linq transformation sample xml xelement.parse used
XElement project = XElement.Parse (@"
<Project DefaultTargets=""Build"" xmlns=""http://schemas.microsoft.com/developer/msbuild/2003"">
<PropertyGroup>
<Platform Condition="" '$(Platform)' == '' "">AnyCPU</Platform>
<ProductVersion>9.0.11209</ProductVersion>
</PropertyGroup>
<ItemGroup>
<Compile Include=""ObjectGraph.cs"" />
<Compile Include=""Program.cs"" />
<Compile Include=""Properties\AssemblyInfo.cs"" />
<Compile Include=""Tests\Aggregation.cs"" />
<Compile Include=""Tests\Advanced\RecursiveXml.cs"" />
</ItemGroup>
</Project>");
XNamespace ns = project.Name.Namespace;
var query =
new XElement ("ProjectReport",
from compileItem in project.Elements (ns + "ItemGroup").Elements (ns + "Compile")
let include = compileItem.Attribute ("Include")
where include != null
select new XElement ("File", include.Value)
);
query.Dump();
and results will be:
<ProjectReport>
<File>ObjectGraph.cs</File>
<File>Program.cs</File>
<File>Properties\AssemblyInfo.cs</File>
<File>Tests\Aggregation.cs</File>
<File>Tests\Advanced\RecursiveXml.cs</File>
</ProjectReport>
more...
Saturday
wcf 404 not found on .csv file (server 2003) .NET 3.0/3.5
To fix this 404 error when openening .csv file
in browser see
this post:
it has basic steps of wcf (Windows Communication Foundation) dot.NET 3.0/3.5 initialization on IIS 6.
in browser see
this post:
it has basic steps of wcf (Windows Communication Foundation) dot.NET 3.0/3.5 initialization on IIS 6.
Friday
get last date of month - csharp
private DateTime LastDayOfMonth(DateTime d)
{
DateTime d = new DateTime(d.Year, d.Month, 1);
return d.AddMonths(1).AddDays(-1);
}
more...
register wcf service on iis by servicemodelreg.exe
I reinstalled aspnet (just in case) this step may be omited:
1.Run service modeling utility: ServiceModelReg.exe /i /x
2. run it again with this key: ServiceModelReg.exe /s:W3SVC
As optional you may refresh this :
c:\WINDOWS\Microsoft.NET\Framework\v3.5\WFServicesReg.exe /r
c:\WINDOWS\Microsoft.NET\Framework\v3.5\WFServicesReg.exe /c
http://social.msdn.microsoft.com/Forums/en-US/wcf/thread/95bdf2fa-3e2d-489d-8873-31f0da816577/
more...
C:\WINDOWS\Microsoft.NET\Framework\v2.0.50727>aspnet_regiis.exe -i
Start installing ASP.NET (2.0.50727).
..........................
Finished installing ASP.NET (2.0.50727).
1.Run service modeling utility: ServiceModelReg.exe /i /x
C:\WINDOWS\Microsoft.NET\Framework\v3.0\Windows Communication Foundation>ServiceModelReg.exe /i /x
Microsoft(R) Windows Communication Foundation Installation Utility
[Microsoft (R) Windows (R) Communication Foundation, Version 3.0.4506.2152]
Copyright (c) Microsoft Corporation. All rights reserved.
Installing: Machine.config Section Groups and Handlers
Installing: System.Web Build Provider
Installing: System.Web Compilation Assemblies
Installing: HTTP Handlers
Installing: HTTP Modules
Installing: Web Host Script Mappings
Installing: WMI Classes
Installing: Windows CardSpace (idsvc)
Installing: Net.Tcp Port Sharing Service (NetTcpPortSharing)
Installing: HTTP Namespace Reservations
2. run it again with this key: ServiceModelReg.exe /s:W3SVC
C:\WINDOWS\Microsoft.NET\Framework\v3.0\Windows Communication Foundation>Service
ModelReg.exe /s:W3SVC
Microsoft(R) Windows Communication Foundation Installation Utility
[Microsoft (R) Windows (R) Communication Foundation, Version 3.0.4506.2152]
Copyright (c) Microsoft Corporation. All rights reserved.
Installing: Web Host Script Mappings
As optional you may refresh this :
c:\WINDOWS\Microsoft.NET\Framework\v3.5\WFServicesReg.exe /r
c:\WINDOWS\Microsoft.NET\Framework\v3.5\WFServicesReg.exe /c
http://social.msdn.microsoft.com/Forums/en-US/wcf/thread/95bdf2fa-3e2d-489d-8873-31f0da816577/
more...
Thursday
add google search to website
To add google search box please copy code below. (replace sourcefield.blogspot.com with your address)
Also you can get free virus protection from google within google pack.
Also you can get free virus protection from google within google pack.
<input class="BlogSearch" type="text" name="searchBox" id="blogSearchText" value="" onkeypress="return blogSearch(event, this);">
<input type="button" value="Search" onclick="return blogSearch2('blogSearchText');" class="BlogSearchButton">
<script type="text/javascript">
function blogSearch(event, oInput) {
var keyCode = (event) ? event.keyCode : keyStroke.which;
if (keyCode == 13) {
top.location = 'http://www.google.com/search?q=' + escape(oInput.value) + '+site%3Asourcefield.blogspot.com';
return false;
} return true;
}
function blogSearch2(oInputId) {
var oInput = document.getElementById(oInputId);
top.location = 'http://www.google.com/search?q=' + escape(oInput.value) + '+site%3Asourcefield.blogspot.com';
return false;
}
</script>
where to buy visual studio 2008
I downloaded Free 90-Day Trial from Microsoft and was defying all challenges with success :).
Now time has come today to buy, so I was looking for deals on studio in the market.
There is a lot academic versions software for students on "Academic superstore" for teachers students and schools.
First obvious thing I can buy standard edition or professional edition on Amazon, they have free shipping,
but there are a lot of other hot deals as well, let's compare prices:
Visual.EverythingOutlet from $149
SoftwareKing - from $149
AcademicSuperstore - from $195
Amazon - 249.99
buypcsoft has it from $679.95
I'm still looking for visual studio so if anybody did it , please share your experience.
more...
Now time has come today to buy, so I was looking for deals on studio in the market.
There is a lot academic versions software for students on "Academic superstore" for teachers students and schools.
First obvious thing I can buy standard edition or professional edition on Amazon, they have free shipping,
but there are a lot of other hot deals as well, let's compare prices:
Visual.EverythingOutlet from $149
SoftwareKing - from $149
AcademicSuperstore - from $195
Amazon - 249.99
buypcsoft has it from $679.95
I'm still looking for visual studio so if anybody did it , please share your experience.
more...
linq examples in linqpad
just downloaded linqpad, it contains a lot of examples linq to sql from C# 3.0 in a Nutshell book.
including linq grouping and linq join ,nice linq tutorial that allows to run your own linq queries.
more...
including linq grouping and linq join ,nice linq tutorial that allows to run your own linq queries.
more...
Monday
linq transactions support/Charp/dot.net 3.5
[Test]
public void StoredProcedure_and_LinqUpdates_Test()
{
// dx is datacontext instance
dx.Connection.Open();
dx.Transaction = dx.Connection.BeginTransaction();
// calling stored procedure from linq here
var result = dx.PaymentServices_ApplyPaymentToAccount(89);
// updating objects from context
Invoice i = customerService.getInvoice(277);
i.DueDate = Convert.ToDateTime("11/5/2008");
// submitting changes and committing transaction
dx.SubmitChanges();
dx.Transaction.Commit();
// rollback transactions and
// no changes made in object or by stored procedure will be stored
dx.Transaction.Rollback();
}
more...
Friday
remove namespace xml in serialisation by XmlSerializerNamespaces.dot.net/C#/csharp
public static string toXML(Object obj)
{
XmlSerializer xmlSerializer = new XmlSerializer(obj.GetType());
StringWriter stringWriter = new StringWriter();
XmlWriterSettings writerSettings = new XmlWriterSettings();
writerSettings.OmitXmlDeclaration = true;
writerSettings.Indent = true;
using (XmlWriter xmlWriter = XmlWriter.Create(stringWriter, writerSettings))
{
// Namespace removed in these two lines:
XmlSerializerNamespaces ns = new XmlSerializerNamespaces();
ns.Add("", "");
xmlSerializer.Serialize(xmlWriter, obj,ns);
}
return stringWriter.ToString();
}
more...
List XMl serialization/deserialization
To serialize list by using class in previous post :
But for deserialization it'e required to use different method:
sample of the call:
more...
List<DefaultFeeForNewInvoice> fees = new List<DefaultFeeForNewInvoice>();
fees.Add(new DefaultFeeForNewInvoice() { FeeAmount = (decimal)4.5, FeeSubTypeID = 13, PaymentSourceID = 4 });
fees.Add(new DefaultFeeForNewInvoice() { FeeAmount = (decimal)4.5, FeeSubTypeID = 13, PaymentSourceID = 4 });
fees.Add(new DefaultFeeForNewInvoice() { FeeAmount = (decimal)4.5, FeeSubTypeID = 13, PaymentSourceID = 4 });
string str=CXMLConv.toXML(fees);
But for deserialization it'e required to use different method:
/// <summary>
/// Deserialize List dotnet/csharp
/// fees_con = (List<DefaultFeeForNewInvoice>) CXMLConv.Load(str_con, fees_con.GetType(), "ArrayOfDefaultFeeForNewInvoice");
/// </summary>
/// <param name="XMLString">The XML string.</param>
/// <param name="t">The type</param>
/// <param name="RootElementName">Name of the root element.</param>
/// <returns></returns>
static public object Load(string XMLString, Type t, string RootElementName)
{
XmlSerializer mySerializer =
new XmlSerializer(t, new XmlRootAttribute(RootElementName));
StringReader myReader =
new StringReader(XMLString);
return mySerializer.Deserialize(myReader);
}
sample of the call:
fees_con = (List<DefaultFeeForNewInvoice>) CXMLConv.Load(str_con, fees_con.GetType(), "ArrayOfDefaultFeeForNewInvoice");
more...
Dot.NET webservice to enable remote invoke/testing add to web.config under system.web
<system.web>
<webServices>
<protocols>
<add name="HttpPost" />
<add name="HttpGet" />
</protocols>
</webServices>
more...
xml serializer for xml serialization in c# (dot.net v3.5)
public class CXMLConv
{
public static T fromXML<T>(string x, T obj)
{
try {
XmlSerializer xmlSerializer = new XmlSerializer(obj.GetType());
StringReader stringReader = new StringReader(x);
return (T)xmlSerializer.Deserialize(stringReader);
}
catch (Exception ex) {
Logger.prn("Deserializing problem for XML:"+x,ex);
Assertions.Throw("Incorrect incoming XML specified." );
return obj;
}
}
public static string toXML(Object obj)
{
XmlSerializer xmlSerializer = new XmlSerializer(obj.GetType());
StringWriter stringWriter = new StringWriter();
XmlWriterSettings writerSettings = new XmlWriterSettings();
writerSettings.OmitXmlDeclaration = true;
writerSettings.Indent = true;
using (XmlWriter xmlWriter = XmlWriter.Create(stringWriter, writerSettings))
{
xmlSerializer.Serialize(xmlWriter, obj);
}
return stringWriter.ToString();
}
}
more...
linq serialize xml and deserialize into IEnumerable objects ( XMLSerializer class on top post)
public class ProcessingFeeRuleProperty
{
public int FeeID;
public decimal FeeAmount;
}
[TestFixture]
public class UnitTest1
{
public UnitTest1()
{ }
[Test]
public void TestMethod2()
{
ProcessingFeeRuleProperty pr = new ProcessingFeeRuleProperty() {FeeID = 1, FeeAmount = 12};
ProcessingFeeRuleProperty pr2 = new ProcessingFeeRuleProperty() {FeeID = 2, FeeAmount = 24};
//Logger.prn(CXMLConv.toXML(pr));
List<ProcessingFeeRuleProperty> l=new List<ProcessingFeeRuleProperty>();
l.Add(pr);l.Add(pr2);
IEnumerable<ProcessingFeeRuleProperty> p = l;
Logger.prn(CXMLConv.toXML(p));
string xml_str =@"<ArrayOfProcessingFeeRuleProperty >
<ProcessingFeeRuleProperty>
<FeeID>1</FeeID>
<FeeAmount>12</FeeAmount>
</ProcessingFeeRuleProperty>
<ProcessingFeeRuleProperty>
<FeeID>2</FeeID>
<FeeAmount>24</FeeAmount>
</ProcessingFeeRuleProperty>
</ArrayOfProcessingFeeRuleProperty>
";
ProcessingFeeRuleProperty p2 = new ProcessingFeeRuleProperty();
CXMLConv.fromXML<IEnumerable<ProcessingFeeRuleProperty>>(xml_str,p);
foreach (var c in p) {
Logger.prn(c.FeeID.ToString(), c.FeeAmount.ToString());
}
var sel_fee = from x in p
where x.FeeID == 1
select x;
ProcessingFeeRuleProperty first= sel_fee.First();
Logger.prn("\n\n\n",first.FeeID.ToString(), first.FeeAmount.ToString());
}
XMLSerializer class on upper post
more...
Tuesday
linq group by sum and join example
var fees =
from p in i.AccountFees
group p by p.FeeID into g
select new { FeeID = g.Key, TotalAmount = g.Sum(p => p.Amount) };
foreach (var fee in fees) {
Logger.prn("" + fee.FeeID + ":" + fee.TotalAmount);
}
more...
Thursday
python sum of list/array and any all
::accumulators
def countall(it):return sum(1 for x in it )
if any(x>5 for x in xs): ...
if all(x>y>z for x,y,z in zip(xs,ys,ys)):
from itertools import izip
if all(x>y>z for x,y,z in izip(xs,ys,zs)):
print max(i for i, x in enumerate(xs) if x > 0)
with operator:
with open('myfile.txt') as f:
for aline in f: print alien[3]
more...
python containers:
tuple - immutable sequence : (2,3) tulpe ('ciao')
list - mutable sequence: [2,3] list('ciao')
set / frozenset : set() set ((23,)) set ('ciao')
dict map key-value: {2:3}, {4:5,6:7} dict(ci='oo')
All Containers support :
len(c)
iteration : for x in c
membership: if x in c
more...
python help function/command
to get list of all methods/properties use :
>>dir ('mystring')
to get help for 'center' method of string we use :
>>help(''.center)
more...
linq sum example / csharp
var activefees = from af in invoice.AccountFees
where af.StatusID == EnumStatusType.Open
select af;
return activefees.Sum(af => af.Amount);
// or more complicated with condition
decimal am = 0;
foreach (XAttribute v in rs.RuleAccountFeesToBill(customer_id)){
long f = long.Parse(v.Value);
am += activefees.Sum(acf => acf.AccountFeeSubType.AccountFeeType.FeeTypeID == f ? acf.Amount : 0 );
}
more...
Wednesday
linq to xml example:
using System.Linq;
using System.Xml.Linq;
[Test]
public void TestMethod1()
{
string xml_str = "<BillingTypes> <BillingType ID=\"1\" Description=\"Statement\" /> <BillingType ID=\"2\" Description=\"Coupon\" /> <BillingType ID=\"3\" Description=\"EFT\" /> <BillingType ID=\"4\" Description=\"Visa\" /> </BillingTypes>";
XDocument xml = XDocument.Parse(xml_str);
var allowed = from billingtype in xml.Elements("BillingTypes").Elements("BillingType")
where billingtype.Attribute("ID").Value == "3"
select billingtype;
foreach (var element in allowed){
Debug.Print(element.Attribute("Description").Value);
}
}
more...
linq example - use where for datacontext object
LinqDataObjectsDataContext dc = new LinqDataObjectsDataContext();
Invoice ret = dc.Invoices.Where(e => e.InvoiceID == id).SingleOrDefault();
more...
datacontext linq refresh example C# - linq example how to save object in linq to sql
public string SaveInvoiceXML(string sInvoice){
try{
Invoice inv = new Invoice();
inv = CXMLConv.fromXML<Invoice>(sInvoice, inv);
LinqDataObjectsDataContext dc = new LinqDataObjectsDataContext();
dc.Invoices.Attach(inv);
dc.Refresh(RefreshMode.KeepCurrentValues, inv);
dc.SubmitChanges();
return OK(CXMLConv.toXML(inv));
}
catch (Exception ex)
{
return RegisterError(ex, "SaveInvoiceXML", sInvoice);
}
}
more...
Linq to SQL C# linq join example
try{
LinqDataObjectsDataContext dc = new LinqDataObjectsDataContext();
/* select * from invoice inner join AccountInvoices on
* invoice.cust= AccountInvoices.cust and invoice.sub = AccountInvoices.sub
*/
StringBuilder sb = new StringBuilder();
sb.AppendFormat("<Invoices customer='{0}' sub='{1}'>", cust, sub);
var ret = from invoice in dc.Invoices
join accountInvoice in dc.AccountInvoices on
invoice.InvoiceID equals accountInvoice.InvoiceID
where accountInvoice.SubID ==sub && accountInvoice.CustomerID ==cust
select invoice;
foreach (Invoice i in ret){
sb.Append(CXMLConv.toXML(i));
}
sb.AppendLine("</Invoices>");
return OK(sb.ToString());
} catch (Exception ex) {
return RegisterError(ex, "getInvoicesXML","cust/sub"+cust+"/"+sub);
}
more...
Tuesday
to fix circular reference was detected while serializing an object:
in a c# - in all sub classes find reference to parent class and add
[XmlIgnore] attribute.
Bad news is that you have to do that for all objects every time dbml file was changed.
So I have create simple python script that do this for me:
linesToPatch.txt - is simple text file that contains all lines those must have XMLIgnore, for example:
[XmlIgnore] attribute.
Bad news is that you have to do that for all objects every time dbml file was changed.
So I have create simple python script that do this for me:
import os
import re
file_to_patch='LinqDataObjects.designer.cs'
bak_file='LinqDataObjects.designer.cs.bak'
os.rename(file_to_patch,bak_file)
fileHandle = open ('linesToPatch.txt')
linesToPatch = fileHandle.readlines()
f = open(bak_file)
new_file=open(file_to_patch, 'w')
new_file.write("using System.Xml.Serialization;\n")
for aline in f:
new_file.write(aline)
for rline in linesToPatch:
if aline.strip() == rline.strip():
new_file.write("\t[XmlIgnore]\n")
break
linesToPatch.txt - is simple text file that contains all lines those must have XMLIgnore, for example:
[Association(Name="Invoice_AccountFee", Storage="_Invoice", ThisKey="InvoiceID", OtherKey="InvoiceID", IsForeignKey=true)]
[Association(Name="Accounts_basic_AccountInvoice", Storage="_Accounts_basic", ThisKey="CustomerID,SubID", OtherKey="customer_,sub", IsForeignKey=true)]
[Association(Name="Invoice_AccountInvoice", Storage="_Invoice", ThisKey="InvoiceID", OtherKey="InvoiceID", IsForeignKey=true)]
[Association(Name="AccountFeeType_AccountFeeSubType", Storage="_AccountFeeSubTypes", ThisKey="FeeTypeID", OtherKey="stFeeTypeID")]
Saturday
workout heart rates calculation
1. Calculate maximum hear rate for your age : MHR=214-(0.8 * Age)
example: for 33 years it will be 187.6 per min or 31.2 for ten seconds.
2.Comfort range must be between 60%-75% of maximum hear rate :
between MHR*0.6 and MHR*0.75 per minute or
MHR *0.6/6 and MHR*0.75/6
example: for 33 years it will be between 112.5 - 140.7 per minute or
between 18.75-23.45 per 10 seconds
count pulse during 10 second and make sure you are in comfort zone.
example:If you are 33 you must have from 18 to 23 pulses for 10 second.
more...
Friday
to fix wcf soap client error :
WSDLService :Processing service xxx found no port definitions HRESULT=0x80070057: The parameter is incorrect.
update web.config:
...
basicHttpBinding is compatible with old soap clients.
more...
update web.config:
...
<services>
<service name="Business.WebService.Service1" behaviorConfiguration="Business.WebService.Service1Behavior">
<!-- Service Endpoints -->
find this line: <endpoint address="" binding="wsHttpBinding" contract="Business.WebService.IService1">
and replace with this one : <endpoint address="" binding="basicHttpBinding" contract="Business.WebService.IService1">
basicHttpBinding is compatible with old soap clients.
more...
Tuesday
javascript transformnode or javascript transform xml
XSL transformation in javascript
// ake xmldoc for client -side transformations
function MakeXMLDoc()
{
var xmlDoc;
// code for IE
if (window.ActiveXObject){
xmlDoc=new ActiveXObject("Microsoft.XMLDOM");
}
// code for Mozilla, Firefox, Opera, etc.
else if (document.implementation && document.implementation.createDocument) {
xmlDoc=document.implementation.createDocument("","",null);
}else{
alert('Your browser cannot handle this script');
}
xmlDoc.async=false;
return(xmlDoc);
}
function XSLTrasformer(xml,xsl,span_name) // >[:>]
{
//xml=loadXMLDoc("1.xml");
//xsl=loadXMLDoc("NovaSettleResponse.xsl");
// code for IE
if (window.ActiveXObject)
{
ex=xml.transformNode(xsl);
document.getElementById(span_name).innerHTML=ex;
}
// code for Mozilla, Firefox, Opera, etc.
else if (document.implementation
&& document.implementation.createDocument)
{
xsltProcessor=new XSLTProcessor();
xsltProcessor.importStylesheet(xsl);
resultDocument = xsltProcessor.transformToFragment(xml,document);
document.getElementById(span_name).appendChild(resultDocument);
}
}
function XMLPopup(xml_string,xsl_file){
xsl=MakeXMLDoc(); xml=MakeXMLDoc();
xsl.load(xsl_file);
xml.loadXML(xml_string);
ex=xml.transformNode(xsl);
//document.getElementById(span_name).innerHTML=ex;
var dbgwin;
//left=0,top=0,
dbgwin=window.open("", "xml_pop", "width=420,height=540,scrollbars=yes,status=yes,resizable=yes");
dbgwin.document.write(ex);
dbgwin.document.write("<p><input type='button' onclick='window.close();' value='close'></p>");
}
call web service from classic asp or vb6 (SOAP Toolkit 3.0)
1.download and install SOAP Toolkit 3.0 from microsoft website
2.here is sample of calling asp.net web service from classic asp:
on this sample XML returning from one of services methods will transformed by xslt and writed on page.
more...
2.here is sample of calling asp.net web service from classic asp:
on this sample XML returning from one of services methods will transformed by xslt and writed on page.
<%
Dim SoapClient
Application.Lock
Set SoapClient = Server.CreateObject("MSSOAP.SoapClient30")
SoapClient.ClientProperty("ServerHTTPRequest") = True
'production url
SoapClient.MSSoapInit "https://services.server.com/clientalerts/alert.asmx?WSDL"
'add
Set Application("CalcRpcAspVbsClient") = SoapClient
Application("CalcRpcAspVbsClientInitialized") = True
Application.UnLock
'call as usual
Response.Write SoapClient.Transformdata("c:\xslt\setup.xslt", SoapClient.getSubscriptions(Session("DealerId")))%>
more...
System.Web.HttpException: Maximum request length exceeded
to fix this exception add line to web.config :
Transferring large objects over webservice (MSDN)
more...
<httpRuntime maxRequestLength="81920" executionTimeout="900" />
</system.web>
</configuration>
Transferring large objects over webservice (MSDN)
more...
Monday
System.Net.WebException: The underlying connection was closed: Could not establish trust relationship for the SSL/TLS secure channel.
[Web Service in .net , webservice security]
when call webservice with bad certificate (VB.NET)
more...
when call webservice with bad certificate (VB.NET)
1.Add imports
Imports System.Net.Security
Imports System.Security.Cryptography.X509Certificates
2.before calling webservice:
ServicePointManager.ServerCertificateValidationCallback = New RemoteCertificateValidationCallback(AddressOf customXertificateValidation)
3.Custom certificate runner:
Private Shared Function customXertificateValidation(ByVal sender As Object, ByVal cert As X509Certificate, ByVal chain As X509Chain, ByVal Errora As SslPolicyErrors) As Boolean
Return True
End Function
more...
Saturday
Autojump: Jumping game script : autohotkey
SetKeyDelay, 75, 75
;Exits AutoHotKey application.
$^CapsLock::
ExitApp
return
;Pauses AutoHotKey Script.
F6::Pause, Toggle, 1
$x::
Loop {
Send, {Space}
sleep 60
Send, {Space}
sleep 60
Send, {Space}
sleep 60
Send, {Space}
}
more...
Wednesday
Ho wto fix "the definition of object * has changed since it was compiled." error:
run fololowing :
exec sp_recompile sp_your_name
OUTPUT:
Object 'sp_yourname' was successfully marked for recompilation.
more...
exec sp_recompile sp_your_name
OUTPUT:
Object 'sp_yourname' was successfully marked for recompilation.
more...
Tuesday
make xsd from xml (C#/csharp)
[Test]
public void generateXsd() {
DataSet d = new DataSet();
d.ReadXml(@"C:\projects\LeadInformation.xml");
d.WriteXmlSchema(@"C:\projects\LeadInformation.xsd");
}
more...
Thursday
grep how to find recursively all files with keywords from keyword file
assume that kw.txt is text file with keywords we have to find , then :
grep -irn -f ../kw.txt . *
more...
grep -irn -f ../kw.txt . *
more...
TSQL:MS SQL SERVER:Find stored procedure by keyword or column
SELECT sys.sysobjects.name, sys.syscomments.text
FROM sys.sysobjects INNER JOIN syscomments
ON sys.sysobjects.id = sys.syscomments.id
WHERE sys.syscomments.text LIKE '%single_pay_renew%'
AND sys.sysobjects.type = 'P'
ORDER BY sys.sysobjects.NAME
more...
Friday
WINService in python sample
import win32serviceutil
import win32service
import win32event
class SmallestPythonService(win32serviceutil.ServiceFramework):
_svc_name_ = "SmallestPythonService"
_svc_display_name_ = "The smallest possible Python Service"
def __init__(self, args):
win32serviceutil.ServiceFramework.__init__(self, args)
# Create an event which we will use to wait on.
# The "service stop" request will set this event.
self.hWaitStop = win32event.CreateEvent(None, 0, 0, None)
def SvcStop(self):
# Before we do anything, tell the SCM we are starting the stop process.
self.ReportServiceStatus(win32service.SERVICE_STOP_PENDING)
# And set my event.
win32event.SetEvent(self.hWaitStop)
def SvcDoRun(self):
# We do nothing other than wait to be stopped!
win32event.WaitForSingleObject(self.hWaitStop, win32event.INFINITE)
if __name__=='__main__':
win32serviceutil.HandleCommandLine(SmallestPythonService)
more...
Thursday
port forwarding RDP;cannot connect to localhost
symptoms:
"The client could not connect. You
are already connected to the console of this computer. A new console
session cannot be established".
Procedure:
1. Create a folder (For example c:\TSclient) on your XP box
2. Copy mstsc.exe and mstscax.dll from your XP's %systemroot%\system32
to this folder.
3. Right click mstsc.exe and go to the properties of it.
4. Select the Compatibility tab
5. Check "Run this program in compatibility mode for"
6. Select "Windows 98/ Windows Me"
7. Click on OK
8. Connect your SSH to the remote network or machine
9. Forward another port than 3389, for example 3390
10. Use the newly copied mstsc.exe to connect to 127.0.0.1:3390
more...
Monday
Wednesday
simple python unit test sample,TDD rule
import unittest
class TestTank(unittest.TestCase):
def testImage(self):
tank="boo"
assert tank != "boo", "tank image is None"
def test1(self):
tank ="moo"
assert tank != "moo", "test1 tank image is None"
if __name__=="__main__":
unittest.main()
more...
Generate XSD from XML with DataSet (VB.NET)
more...
<Test()> _
Public Sub generateXsd()
Try
Dim d As DataSet = New DataSet()
d.ReadXml("C:\projects\LeadInformation.xml")
d.WriteXmlSchema("C:\projects\LeadInformation.xsd")
Catch ex As Exception
End Try
End Sub
SQL server 2005-create indexes for temporary tables t-sql
1 SELECT
2 ad.[DealerId]
3 ,ad.[APSDealerId]
4 ,cu.CustomerID
5 ,cu.[FirstName]
6 ,cu.[LastName]
7 ,cu.[DOB]
8 ,cu.OwnerID
9 ,cu.referencefield1
10 ,cu.referencefield2
11 into #tmain
12 FROM [Customers] cu
13 INNER JOIN [AdvanceCustomer] ac ON ac.[CustomerID] = cu.[CustomerID]
14 INNER JOIN [ApsUbillDealer] ad ON ad.[DealerId] = ac.[DealerID]
15 WHERE cu.[DateCreated] > @XmlData.value('(/args/FromDate)[1]','varchar(10)')
16 AND cu.[DateCreated] < @XmlData.value('(/args/ToDate)[1]','varchar(10)')
17
18 CREATE UNIQUE CLUSTERED INDEX IX_1 on #tmain (CustomerID, OwnerID)
19 CREATE INDEX IX_2 on #temp_employee_v1 (APSDealerId)
20
21
more...
Thursday
To Add gVIM to External Tools to Visual Studio or SQL Management Studio
Title:vim&-
Command:C:\vim\vim71\gvim.exe
Arguments: -p -o --remote-silent +$(CurLine) $(ItemPath)
now vim can be called by : <Alt-T> -
more...
Command:C:\vim\vim71\gvim.exe
Arguments: -p -o --remote-silent +$(CurLine) $(ItemPath)
now vim can be called by : <Alt-T> -
more...
Monday
Create SQL INSERT from Excel file (ruby script)
import excel file into SQL.
first row is column names.
first row is column names.
require 'win32ole'
xl = WIN32OLE.connect('Excel.Application')
wb = xl.ActiveWorkbook
wb.Worksheets.each do |ws|
data = ws.UsedRange.Value
if data!=nil
field_names = data.shift
flds=field_names.join(',')
data.each do |row|
row.collect! { |f| f = "'" + f.to_s + "'" }
puts ("INSERT INTO [#{ws.Name}] (#{flds}) VALUES \
( #{row.join(',')} );")
end
end
end
As alternative there is app that can convert Excel into SQL witn CREATE TABLE and INSERT statements
Handling custom error messages from sql in VB.NET (System.SslClient , SQL Server 2005)
Imports System.Data.SqlClient
Imports CSharp.Core
Public Class DBProvider
Public Sub LogSQLMessages(ByVal sender As Object, ByVal e As SqlInfoMessageEventArgs)
Logger.prn("SQLMessage:" & e.ToString)
End Sub
Private Shared youBillConn As String = ConfigurationManager.ConnectionStrings("conn").ConnectionString
Public Function ExecuteScalar(ByVal spname As String, ByVal ParamArray sparams() As Object) As Object
Dim sqlc As New SqlConnection(youBillConn)
Try
AddHandler sqlc.InfoMessage, AddressOf LogSQLMessages
'sqlc.FireInfoMessageEventOnUserErrors = True
sqlc.Open()
Return SqlHelper.ExecuteScalar(sqlc, spname, sparams)
Catch ex As SqlClient.SqlException
Dim erix As Integer = ex.Message.IndexOf("MSIEX:[[")
Dim eree As Integer = ex.Message.IndexOf("]]")
If erix >= 0 And eree > 0 And eree > erix Then
Assertions.Throw(ex.Message.Substring(erix + 8, (eree - erix) - 8))
Else
Logger.prn(ex)
Throw ex
End If
Return Nothing
Catch allex As Exception
Logger.prn(allex)
Throw allex
Finally
sqlc.Close()
End Try
End Function
End Class
more...
Friday
TRY..CATCH in T-SQL (sqlserver 2005)
SELECT * FROM sys.messages
ALTER Procedure usp_sp1
@XMLData XML
AS
BEGIN
DECLARE
...declarations...
SET XACT_ABORT ON
BEGIN TRY
BEGIN TRANSACTION usp_sp1_tran
...transactions...
COMMIT TRANSACTION usp_sp1_tran
END TRY
BEGIN CATCH
ROLLBACK TRAN usp_sp1_tran
DECLARE @ErrorMessage NVARCHAR(4000)
,@ErrorNumber INT
,@ErrorSeverity INT
,@ErrorState INT
,@ErrorLine INT
,@ErrorProcedure NVARCHAR(200) ;
SELECT @ErrorNumber = ERROR_NUMBER()
,@ErrorSeverity = ERROR_SEVERITY()
,@ErrorState = ERROR_STATE()
,@ErrorLine = ERROR_LINE()
,@ErrorProcedure = ISNULL(ERROR_PROCEDURE(), '-') ;
SELECT @ErrorMessage = 'Procedure:' + @ErrorProcedure + '; Line:'
+ CAST(@ErrorLine AS VARCHAR(10)) + '; ' + ERROR_MESSAGE() ;
--EXEC usp_Email @ErrorMessage, 'mymail@host.com'
RAISERROR ( @ErrorMessage, 16, 1 )
END CATCH
END
more...
Custom RAISERROR in SQL Server 2005 (T-SQL)
how to add custom message %s is place holder for argument:
to see your message in messages table:
Assert procedure in TSQL :
this setting terminate stored procedure after RAISERROR
more...
exec sp_addmessage @msgnum = 60555, @severity = 16,
@msgtext = 'MSIEX:[[%s]]',
@lang = 'us_english';
go
to see your message in messages table:
SELECT * FROM sys.messages
Assert procedure in TSQL :
ALTER PROC usl_AssertionsThrow
@msg VARCHAR(MAX)
AS
BEGIN
RAISERROR ( 60555, 20, 1,@msg) WITH LOG
END
this setting terminate stored procedure after RAISERROR
SET XACT_ABORT ON
more...
Subscribe to:
Posts (Atom)
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
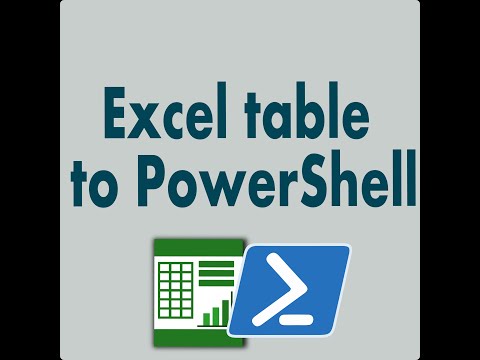
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
2010-11-24 Update: Please download latest version of Pidgin , that has this problem fixed , no additional steps required. Here are 3 ways to...