Wednesday
sloniki
Thursday
script create table
declare @table varchar(100)
set @table = 'MyTable' -- set table name here
declare @sql table(s varchar(1000), id int identity)
-- create statement
insert into @sql(s) values ('create table [' + @table + '] (')
-- column list
insert into @sql(s)
select
' ['+column_name+'] ' +
data_type + coalesce('('+cast(character_maximum_length as varchar)+')','') + ' ' +
case when exists (
select id from syscolumns
where object_name(id)=@table
and name=column_name
and columnproperty(id,name,'IsIdentity') = 1
) then
'IDENTITY(' +
cast(ident_seed(@table) as varchar) + ',' +
cast(ident_incr(@table) as varchar) + ')'
else ''
end + ' ' +
( case when IS_NULLABLE = 'No' then 'NOT ' else '' end ) + 'NULL ' +
coalesce('DEFAULT '+COLUMN_DEFAULT,'') + ','
from information_schema.columns where table_name = @table
order by ordinal_position
-- primary key
declare @pkname varchar(100)
select @pkname = constraint_name from information_schema.table_constraints
where table_name = @table and constraint_type='PRIMARY KEY'
if ( @pkname is not null ) begin
insert into @sql(s) values(' PRIMARY KEY (')
insert into @sql(s)
select ' ['+COLUMN_NAME+'],' from information_schema.key_column_usage
where constraint_name = @pkname
order by ordinal_position
-- remove trailing comma
update @sql set s=left(s,len(s)-1) where id=@@identity
insert into @sql(s) values (' )')
end
else begin
-- remove trailing comma
update @sql set s=left(s,len(s)-1) where id=@@identity
end
-- closing bracket
insert into @sql(s) values( ')' )
-- result!
select s from @sql order by id
Tuesday
postback using javascript
<asp:UpdatePanel runat="server">
<ContentTemplate>
<div>
<asp:Literal runat="server" ID="ChildWindowResult" />
</div>
<hr />
<input type="button" value="Open Dialog" onclick="window.open('MyDialog.aspx', 'Dialog');" />
<asp:Button ID="HiddenButtonForChildPostback" runat="server"
OnClick="OnChildPostbackOccured" style="display: none;" />
<asp:HiddenField runat="server" ID="PopupWindowResult"/>
</ContentTemplate>
</asp:UpdatePanel>
The MyDialog page:
<script type="text/javascript" src="http://ajax.aspnetcdn.com/ajax/jQuery/jquery-1.6.1.min.js"></script>
<script type="text/javascript">
function postData() {
var resultField = $("input[type='hidden'][id$='PopupWindowResult']", window.opener.document);
var parentPosDataButton = $("[id$='HiddenButtonForChildPostback']", window.opener.document);
resultField.val($("#<%= SomeValueHiddenField.ClientID %>").val());
parentPosDataButton.click();
}
</script>
<asp:TextBox runat="server" ID="SomeValueHiddenField" />
<asp:Button runat="server" OnClick="PostData" Text="Click Me" />
in code behind class:
protected void PostData(object sender, EventArgs e)
{
SomeValueHiddenField.Value = DateTime.Now.ToString();
ClientScript.RegisterStartupScript(this.GetType(), "PostData", "postData();", true);
}
postback example in asp.net
// On the button control, inside the event
// Check if indicating to continue or intercept, open the popup,
// and define the callback with the "sender" (the button itself)
if (!(bool)Session["IsAllowed"])
{
// Open popup to enter the password, and define a callback
Page.RegisterStartupScript(
"openwindow", "<script>window.open('http://myurl.aspx'" +
",'title');" +
"myPage_Callback = function(){" +
Page.GetPostBackEventReference((System.Web.UI.Control)sender,"") +
"};" +
"</script>"
);
return;
}
// On popup window, on event of the submit button
// Calls the __doPostBack callback js function on the opener and closes the popup.
Session["IsAllowed"] = true;
Page.RegisterStartupScript(
"closewindow", "<script>window.opener.window.myPage_Callback();" +
"window.close();" +
"</script>"
);
Friday
scrum google spreadsheet
- A simple example (Product
Backlog, Sprint Backlog and Burndown
Charts) provided by Pyxis. - A sample sheet (Product Backlog, Sprint Backlog, Burndown Charts, Impediments backlog) provided by Openbravo - and some more sheets at the end of this page.
- A basic Scrum template including a product backlog and sprint backlogs.
- A Scrum template inspired from Bas Vodde (see this blog post).
- A very simple starting-point for a scrum product backlog.
Thursday
Total commander and Winmerge
CompareTool="d:\Program Files\WinMerge\WinMerge.exe"
html5 mobile framework
Titanium -Appcelerator Titanium is a free and open source framework to develop easily native mobile and desktop apps with web technologies. It provides developers with over 100 customizable UI controls for native tables, views, tabs, alerts, dialogs, buttons, support for geolocation, social networks and multimedia.
Sencha Touch -Sencha Touch is a HTML5 mobile app framework that allows you to develop web apps that look and feel native on Apple iOS and Google Android touchscreen devices. It supports HTML5, CSS3, and Javascript for the highest level of power, flexibility, and optimization in developing your web applications.
JQTouch - (JQtouch has now moved on to Sencha Touch )- A jQuery plugin for mobile web development on the iPhone, Android, iPod Touch, and other forward-thinking devices.
Sproutcore Touch -Sproutcore Touch is the touch edition of the Sproutcore framework for developing HTML 5 web applications that includes complete support for touch events and hardware acceleration on the iPad and iPhone.
PhoneGap -PhoneGap is another interesting open source framework for building cross-platform mobile apps with web standars (HTML5, CSS3, JavaScript). This framework supports geolocation, vibration, accelerometer, camera, orientation change, magnetometer and other interesting features for iPhone, Android, Blackberry, Symbian and Palm.
Rhodes - Rhodes is another excellent open source framework to rapidly build native apps for all major smartphone operating systems (iOS, Windows Mobile, Symbian and Android). It supports GPS geolocation, PIM contact reading and writing, and camera image capture.
iUI -iUI is a framework consisting of a JavaScript library, CSS, and images for developing advanced mobile webapps for iPhone and comparable/compatible devices.
iWebkit -iWebkit 5 is the new version of the popular ultralight framework for easily creating iPhone and iPod touch applications. The current release has new improved features and is really easy to understand in order to develop in just a few minutes your own web apps.
XUI -XUI is another javascript framework for building simple web applications for mobile devices. No much documentation available but it worth to try it for not complex apps.
jQPad -jQPad is an iPad web development framework jQuery based with some features for quickly developing simple iPad applications.
jQuery Mobile -Closing I want to suggest you jQuery Mobile, the touch-optimized version of the popular jQuery framework for smartphones and tablets which will allow you to design a single highly branded and customized web application that will work on all popular smartphone and tablet platforms. The framework will support iOs, Android, Windows Phone, BlackBerry, Symbian, Palm webOS and other devices. The framework is under development and will be available in late 2010.
foneFrame - foneFrame Mobile Web Framework HTML5 CSS3 Mobile Template
preventing double form submission
btnSubmit.Attributes.Add("onclick", "this.disabled=true;");
Monday
dotnetnuke user control inside user control problem with resx file
public partial class BaseUserControl : DotNetNuke.Entities.Modules.PortalModuleBase
{
// basically this will fix the localization issue
protected override void OnInit(EventArgs e)
{
base.OnInit(e);
string FileName = System.IO.Path.GetFileNameWithoutExtension(this.AppRelativeVirtualPath);
if (this.ID != null)
//this will fix it when its placed as a ChildUserControl
this.LocalResourceFile = this.LocalResourceFile.Replace(this.ID, FileName);
else
// this will fix it when its dynamically loaded using LoadControl method
this.LocalResourceFile = this.LocalResourceFile + FileName + ".ascx.resx";
}
}
Friday
learn mobile development online
The university notes that the two Stanford prerequisite courses, Programming Methodology [Link] and Programming Abstractions [Link], are also available on iTunes U.
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
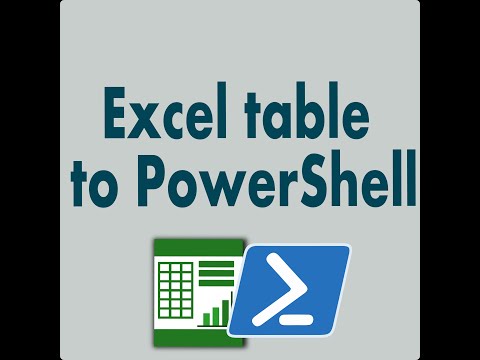
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
2010-11-24 Update: Please download latest version of Pidgin , that has this problem fixed , no additional steps required. Here are 3 ways to...