EmployeeDetail_Dataset.WriteXml("c:\temp.xml")
now xml reflecting the dataset will be in c:\temp.xml
more...
Wednesday
dot.net application or dot.net dll location VB.NET/C#
here's few ways to get application/dot net dll location :
System.AppDomain.CurrentDomain.BaseDirectory
System.AppDomain.CurrentDomain.SetupInformation.ApplicationBase
for ASP.NET u can use following :
Server.MapPath(".")
more...
System.AppDomain.CurrentDomain.BaseDirectory
System.AppDomain.CurrentDomain.SetupInformation.ApplicationBase
for ASP.NET u can use following :
Server.MapPath(".")
more...
Javascript log/debug window for client-site logging
1.Add this code to page or include
use log('my message') function in javascript to show data in window during javascript execution.
more...
var okno;
okno=window.open("", "Debug", "left=0,top=0,width=300,height=700,scrollbars=yes," +"status=yes,resizable=yes");
function log(msg){
okno.document.write("<br>"+msg);
}
use log('my message') function in javascript to show data in window during javascript execution.
more...
Tuesday
Regular Expressions - Strong Password Validation
string r =
@"(?x)" + // Ignore spaces within regex expression, for readability
@"^" + // Anchor at start of string
@"(?=.* ( \d | \p{P} | \p{S} ))" + // String must contain a digit or punctuation char or symbol
@".{6,}"; // String must be at least 6 characters in length
Console.WriteLine (Regex.IsMatch ("abc12", r));
Console.WriteLine (Regex.IsMatch ("abcdef", r));
Console.WriteLine (Regex.IsMatch ("ab88yz", r));
more...
Grouping with LINQ to SQL filtered
// The following groups purchases by year, then returns only those groups where
// the average purchase across the year was greater than $1000:
from p in Purchases
group p.Price by p.Date.Year into salesByYear
where salesByYear.Average (x => x) > 1000
select new
{
Year = salesByYear.Key,
TotalSales = salesByYear.Count(),
AvgSale = salesByYear.Average(),
TotalValue = salesByYear.Sum()
}
more...
LINQ Join vs SelectMany:what is faster?
Customer[] customers = Customers.ToArray();
Purchase[] purchases = Purchases.ToArray();
var slowQuery =
from c in customers
from p in purchases where c.ID == p.CustomerID
select c.Name + " bought a " + p.Description;
var fastQuery =
from c in customers
join p in purchases on c.ID equals p.CustomerID
select c.Name + " bought a " + p.Description;
slowQuery.Dump ("Slow local query with SelectMany");
fastQuery.Dump ("Fast local query with Join");
more...
Monday
convert sources into html /free
Generate XML with LINQ (C#)
var customers =
new XElement ("customers",
from c in Customers
select
new XElement ("customer", new XAttribute ("id", c.ID),
new XElement ("name", c.Name),
new XElement ("buys", c.Purchases.Count)
)
);
customers.Dump();
more...
command line xml parser ( free, open source)
located @ http://xmlstar.sourceforge.net/
more...
XMLStarlet Toolkit: Command line utilities for XML
Usage: xml [] [ ]
whereis one of:
ed (or edit) - Edit/Update XML document(s)
sel (or select) - Select data or query XML document(s) (XPATH, etc)
tr (or transform) - Transform XML document(s) using XSLT
val (or validate) - Validate XML document(s) (well-formed/DTD/XSD/RelaxNG)
fo (or format) - Format XML document(s)
el (or elements) - Display element structure of XML document
c14n (or canonic) - XML canonicalization
ls (or list) - List directory as XML
esc (or escape) - Escape special XML characters
unesc (or unescape) - Unescape special XML characters
pyx (or xmln) - Convert XML into PYX format (based on ESIS - ISO 8879)
p2x (or depyx) - Convert PYX into XMLare:
--version - show version
--help - show help
Wherever file name mentioned in command help it is assumed
that URL can be used instead as well.
Type: xml--help for command help
XMLStarlet is a command line toolkit to query/edit/check/transform
XML documents (for more information see http://xmlstar.sourceforge.net/)
more...
Sunday
how to change header in blogger
U can create script like that :
more...
<script type="text/javascript">
var container = document.getElementById("header-inner");
container.innerHTML ="http://sourcefield.blogspot.com";
</script>
more...
Monday
Service has zero application (non-infrastructure) endpoints FIX
During installation of the webservice I discovered inresting error:
This is more IIS issue, webserver has default directory that was deleted and currently not exists.
That caused the problem,so after changing default directory all started working.
more...
WebHost failed to process a request.
Sender Information: System.ServiceModel.Activation.HostedHttpRequestAsyncResult/25773083
Exception: System.ServiceModel.ServiceActivationException: The service '/Business/Service.svc' cannot be activated due to an exception during compilation.
The exception message is: Service 'Business.WebService.Service' has zero application (non-infrastructure) endpoints. This might be because no configuration file was found for your application, or because no service element matching the service name could be found in the configuration file, or because no endpoints were defined in the service element..
System.InvalidOperationException: Service 'Business.WebService.Service' has zero application (non-infrastructure) endpoints. This might be because no configuration file was found for your application, or because no service element matching the service name could be found in the configuration file, or because no endpoints were defined in the service element.
at System.ServiceModel.Description.DispatcherBuilder.EnsureThereAreNonMexEndpoints(ServiceDescription description)
at System.ServiceModel.Description.DispatcherBuilder.InitializeServiceHost(ServiceDescription description, ServiceHostBase serviceHost)
at System.ServiceModel.ServiceHostBase.InitializeRuntime()
at System.ServiceModel.ServiceHostBase.OnBeginOpen()
at System.ServiceModel.ServiceHostBase.OnOpen(TimeSpan timeout)
at System.ServiceModel.Channels.CommunicationObject.Open(TimeSpan timeout)
at System.ServiceModel.ServiceHostingEnvironment.HostingManager.ActivateService(String normalizedVirtualPath)
at System.ServiceModel.ServiceHostingEnvironment.HostingManager.EnsureServiceAvailable(String normalizedVirtualPath)
--- End of inner exception stack trace ---
at System.ServiceModel.AsyncResult.End[TAsyncResult](IAsyncResult result)
at System.ServiceModel.Activation.HostedHttpRequestAsyncResult.End(IAsyncResult result)
Process Name: w3wp
Process ID: 1680
This is more IIS issue, webserver has default directory that was deleted and currently not exists.
That caused the problem,so after changing default directory all started working.
more...
Wednesday
vb6 or asp xsl transform (transformNode)
Private Function Transform(xml_string, xsl_file) As String
Dim xsl, XML
Set xsl = CreateObject("Microsoft.XMLDOM")
Set XML = CreateObject("Microsoft.XMLDOM")
xsl.Load (xsl_file)
XML.LoadXml (xml_string)
Transform = XML.transformNode(xsl)
End Function
more...
Friday
LINQ:check if IEnumerable is empty (CSharp/C#)
public static void NonEmpty<T>(IEnumerable<T> source,string m){
if (source == null) Throw(m);
if (!source.Any()) Throw(m);
}
more...
Local sequence cannot be used in LINQ to SQL implementation of query operators except the Contains() operator
System.NotSupportedException: Local sequence cannot be used in LINQ to SQL implementation of query operators except the Contains() operator
Method has no supported translation to SQL
The cause of these errors may be mismatch b/w server and client side properties.
Or b/w properties those have SQL presentation and that hasn't:
private IQueryable<Invoice> AttachInvoices(LinqDataObjectsDataContext dc,string sInvoices) {
List<InvArg> ral = new List<InvArg>();
ral = (List<InvArg>)CXMLConv.Load(sInvoices, ral.GetType(), "ArrayOfInvArg") ;
IEnumerable<InvArg> ra = ral;
var inv = from i in dc.Invoices
join r in ra on i.InvoiceID equals r.InvoiceID
select i;
return inv;
}
must be modified to :
private List<Invoice> AttachInvoices(LinqDataObjectsDataContext dc,string sInvoices) {
List<InvArg> ral = new List<InvArg>();
ral = (List<InvArg>)CXMLConv.Load(sInvoices, ral.GetType(), "ArrayOfInvArg");
long[] s=ral.Select(p => p.InvoiceID).ToArray();
var inv = from i in dc.Invoices
where s.Contains(i.InvoiceID)
select i;
return inv.ToList();
}
more...
Thursday
display html on vb6 form
1.add Microsoft Internet controls to link reference.
2.Place control on form, I used 'Browser' as control name.
use this function to display :
more...
2.Place control on form, I used 'Browser' as control name.
use this function to display :
Private Sub ShowHtml(s As String)
Browser.Navigate ("about:blank")
' Dim doc As IHTMLDocument2
Set boxDoc = Browser.Document
boxDoc.Write (s)
boxDoc.Close
End Sub
more...
play sound in console or bat file
to play sound in console or bat file use this line
sndrec32.exe /play /embedding c:\i386\Blip.wav
more...
sndrec32.exe /play /embedding c:\i386\Blip.wav
more...
Wednesday
run regsvr32 command for all files in folder
you can create simple bat file with one line
--regdir--
for %%f in (%1\*.ocx) do (regsvr32 /s "%%f")
--end of regdir--
then run >: regdir c:\mylibs
that might be useful if you want to run command for all files
more...
--regdir--
for %%f in (%1\*.ocx) do (regsvr32 /s "%%f")
--end of regdir--
then run >: regdir c:\mylibs
that might be useful if you want to run command for all files
more...
Tuesday
allow opening chm file from network drives
add this key to registry, or copy-paste it into reg file execute:
---Begin Reg FILE--
Windows Registry Editor Version 5.00
[HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\HTMLHelp\1.x\ItssRestrictions]
"MaxAllowedZone"=dword:00000001
---End Reg FILE--
more...
---Begin Reg FILE--
Windows Registry Editor Version 5.00
[HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\HTMLHelp\1.x\ItssRestrictions]
"MaxAllowedZone"=dword:00000001
---End Reg FILE--
more...
Monday
Subscribe to:
Posts (Atom)
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
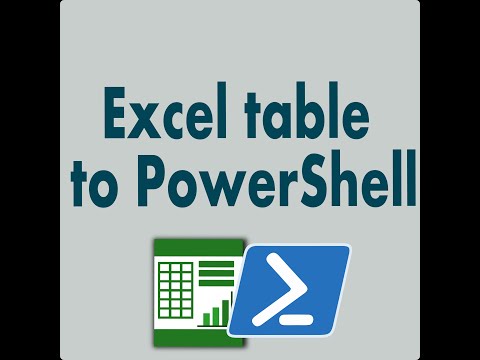
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
2010-11-24 Update: Please download latest version of Pidgin , that has this problem fixed , no additional steps required. Here are 3 ways to...