using System;
using System.Data;
using System.Configuration;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
using System.Reflection;
using System.Collections.Generic;
using System.Linq.Expressions;
public static class Extenders
{
public static List<T> Sort<T>(this List<T> source, string sortExpression)
{
string[] sortParts = sortExpression.Split(' ');
var param = Expression.Parameter(typeof(T), string.Empty);
try
{
var property = Expression.Property(param, sortParts[0]);
var sortLambda = Expression.Lambda<Func<T, object>>(Expression.Convert(property, typeof(object)), param);
if (sortParts.Length > 1 && sortParts[1].Equals("desc", StringComparison.OrdinalIgnoreCase))
{
return source.AsQueryable<T>().OrderByDescending<T, object>(sortLambda).ToList<T>();
}
return source.AsQueryable<T>().OrderBy<T, object>(sortLambda).ToList<T>();
}
catch (ArgumentException)
{
return source;
}
}
}
Sunday
List sorting by SQL ASC/DESC Extender
linq select into class list
let's say we have following class:
and want to create list of these classes , to bind them to grid for example :
public class GridCell {
public string page_name{get;set;}
public string link_code { get; set; }
public int page_state { get; set; }
public int landing_pages_id { get; set; }
}
and want to create list of these classes , to bind them to grid for example :
LandingPagesDataContext lpdc = new LandingPagesDataContext();
var query = from p in lpdc.landing_pages
select new { p.page_name, p.link_code, p.page_state, p.landing_pages_id };
bind(query.Select(p=>new GridCell{page_name = p.page_name, link_code = p.link_code, page_state = p.page_state, landing_pages_id = p.landing_pages_id }).ToList<GridCell>());
Wednesday
find lines from one file into another
How to find lines from one file into another ?
here is sample script showing lines from "find.txt" file those are into "findinto.txt"
common.py find.txt findinto.txt
here is sample script showing lines from "find.txt" file those are into "findinto.txt"
common.py find.txt findinto.txt
import os
import re
import sys
fileHandle = open (sys.argv[1])
linesToPatch = fileHandle.readlines()
f = open(sys.argv[2])
for aline in f:
for rline in linesToPatch:
p=re.compile(rline.strip())
if p.search(aline.strip()):
print aline
Monday
SQL Next business Day Function
-- get next business day
-- assumption: first day of the week is Sunday
CREATE FUNCTION NextBusinessDay
(@date DATETIME)
RETURNS VARCHAR(12)
AS
BEGIN
DECLARE @date1 DATETIME,
@m INT
SELECT @date1 = @date, @m = 0
WHILE @m < 1
BEGIN
SET @date1 = DATEADD(DD,1,@date1)
/* ** the following line checks for non-business days (1 and 7)
** IF your business days are different, change the following line to
** comply with your schedule
*/
IF DATEPART(DW,@date1) NOT IN (1,7)
BEGIN
SET @m = @m + 1
END
END
RETURN CAST(@date1 AS VARCHAR(12))
END
javascript:unescape/escape: decode/encode URL characters
<script type="text/javascript">
alert (unescape('Hello%20World'));
alert (escape('Hello World'));
</script>
Tuesday
Microsoft.AnalysisServices.AdomdClient.AdomdErrorResponseException
When trying to connect to Analysis Services 2005 I was getting this error:
Microsoft.AnalysisServices.AdomdClient.AdomdErrorResponseException: Either the user, NT
AUTHORITY\NETWORK SERVICE, does not have access to ...
I have solved this problem by :
1.logging with "Microsoft SQL Server Management Studion" to Analysis Server
2.expanded "Roles"
3.created new "admin" role with Administration priveleges
4.added "Network Service" as Member of this role.
Microsoft.AnalysisServices.AdomdClient.AdomdErrorResponseException: Either the user, NT
AUTHORITY\NETWORK SERVICE, does not have access to ...
I have solved this problem by :
1.logging with "Microsoft SQL Server Management Studion" to Analysis Server
2.expanded "Roles"
3.created new "admin" role with Administration priveleges
4.added "Network Service" as Member of this role.
Monday
return table from stored procedure
To return table from stored procedure:
declare value as table in sql and fill it from stored procedure by insert..execute like in this code below:
declare value as table in sql and fill it from stored procedure by insert..execute like in this code below:
DECLARE @Accounts1 AS TABLE
(
customer NUMERIC(9, 0),
sub TINYINT,
tp1 VARCHAR(100)
)
insert into @Accounts1
execute usp_AccountDifferentialReportSelector @Did,@CycID
to get data from datasource declared in aspx
public bool OrderExists(int order_index) {
DataView dv1 = (DataView)CategoriesDataSource.Select(new DataSourceSelectArguments());
foreach( DataRow d in dv1.Table.Rows){
if ((int)d["order_index"] == order_index) {
gvMerchantCategoriesLabel.Text = "Update Not Successful, Order Index Already exists";
gvMerchantCategoriesLabel.Style.Add("color", "#FF0000");
return true;
}
}
return false;
}
C# find text in .net grid Rows
public bool OrderExists(int order_index)
{
foreach (GridViewRow r in gvMerchantCategories.Rows)
{
int ord1 = 0;
if (int.TryParse(r.Cells[2].Text, out ord1) && ord1 == order_index)
{
gvMerchantCategoriesLabel.Text = "Update Not Successful, Order Index Already exists";
gvMerchantCategoriesLabel.Style.Add("color", "#FF0000");
return true;
}
}
}
Subscribe to:
Posts (Atom)
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
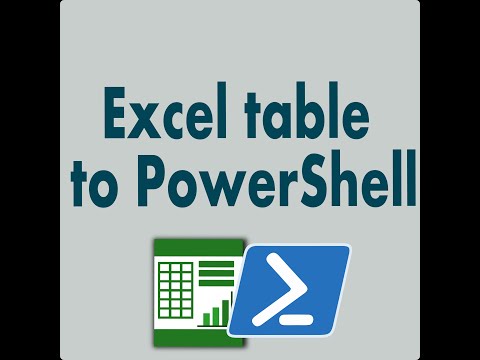
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
2010-11-24 Update: Please download latest version of Pidgin , that has this problem fixed , no additional steps required. Here are 3 ways to...