$('#dvPaymentStatus a:first').text()
Wednesday
jquery get first link in div
this expression returns text of first link in div
jquery all except one
here is jquery how to get all elements except one:
$('#dvPaymentStatus').find("input[type=checkbox]:checked").not('#PaymentStatus_SelectAll').size()
Monday
mvc get html from view
add method below to controller then you will be able to get html from any view by:
this.RenderView("myView");
this.RenderView("myView");
/// <summary>
/// get html from view
/// </summary>
public string RenderView(string ViewName)
{
var content = string.Empty;
var view = ViewEngines.Engines.FindView(ControllerContext, ViewName, null);
using (var writer = new StringWriter())
{
var context = new ViewContext(ControllerContext, view.View, ViewData, TempData, writer);
view.View.Render(context, writer);
writer.Flush();
content = writer.ToString();
}
return content;
}
Wednesday
asp.net mvc ajax post model
If model contain variable of class Class1 with properties Property1,Property2.
Submitted parameters must be named:
"Class1.Property1"
"Class1.Property2"
If model has property as List<Record> submitted values must be :
"Class1[0].Property1"
"Class1[0].Property2"
"Class1[1].Property1"
"Class1[1].Property2"
"Class1[2].Property1"
"Class1[2].Property2"
Here are samples of two functions that creates this values dynamically before submitting form.
Submitted parameters must be named:
"Class1.Property1"
"Class1.Property2"
If model has property as List<Record> submitted values must be :
"Class1[0].Property1"
"Class1[0].Property2"
"Class1[1].Property1"
"Class1[1].Property2"
"Class1[2].Property1"
"Class1[2].Property2"
Here are samples of two functions that creates this values dynamically before submitting form.
//this function create parameters for sending them over AJAX
function ConvertJSONIntoPostableHTML() {
var params = {
param1 : 'myvalue',
};
if (Jsonrecord != null) {
for (var i = 0; i < Jsonrecord.length; i++) {
var rec = Jsonrecord[i];
$.each(Object.keys(rec), function(j, value) {
params["Records[" + i + "]." + value] = rec[value];
});
}
}
return params;
}
//this function add parameters to form , then form can be submitted
function SelectedNodes(frm) {
$(".tslct").remove();
var i = 0;
$.each(myJsonCollection, function () {
var obj = "Nodes[" + i + "].";
addElem(frm, obj + "Id", this.Id);
addElem(frm, obj + "Value", this.Type);
i++;
}
);
}
jquery add element to form dynamically
//function that adds element to form
function addElem(frm, id, val) {
$('<input>').attr({
type: 'hidden',
id: id,
name: id,
value: val,
class: 'tslct'
}).appendTo(frm);
}
//example of using of this function
var frm = $(this).closest('form');
addElem(frm, "myId", "myvalue");
Subscribe to:
Posts (Atom)
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
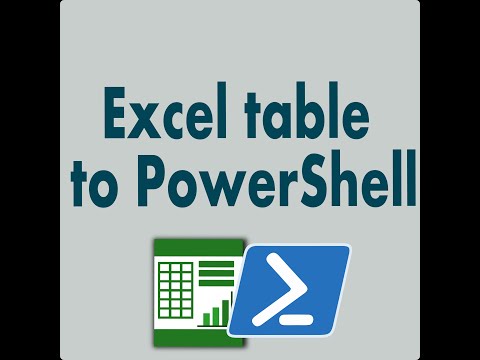
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
2010-11-24 Update: Please download latest version of Pidgin , that has this problem fixed , no additional steps required. Here are 3 ways to...