using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace RulesDemo
{
/* messages */
public class MessageBase
{
public bool ClientActive { get; set; }
}
public class SPQMessage: MessageBase {
public bool Card {get;set;}
}
public class ACPMessage: MessageBase {
public bool URL {get;set;}
}
/* Ruule interface*/
public interface IRule {
string Evaluate (MessageBase messageBase);
string Evaluate (SPQMessage messageBase);
string Evaluate (ACPMessage messageBase);
}
/* rules */
/* rule base */
public class RuleBase: IRule {
public virtual string Evaluate(MessageBase messageBase)
{
throw new NotImplementedException();
}
public virtual string Evaluate(SPQMessage messageBase)
{
return Evaluate((MessageBase)messageBase);
}
public virtual string Evaluate(ACPMessage messageBase)
{
return Evaluate((MessageBase)messageBase);
}
}
/* concrete rules */
public class ClientIsActive : RuleBase
{
public override string Evaluate(MessageBase messageBase)
{
return "ClientIsActive";
}
}
public class UrlIsPresent : RuleBase
{
public override string Evaluate(ACPMessage messageBase)
{
return "UrlIsPresent";
}
}
/* */
class Program
{
static void Main(string[] args)
{
ArrayList list = new ArrayList();
list.Add(new ClientIsActive());
list.Add(new UrlIsPresent());
ACPMessage message = new ACPMessage();
foreach (IRule rule in list)
{
Console.WriteLine(rule.Evaluate(message));
}
/* this will crash because ACP rule doesn't know how to process SPQ message*/
/*
SPQMessage message2 = new SPQMessage();
foreach (IRule rule in list)
{
Console.WriteLine(rule.Evaluate(message2));
}
*/
Console.ReadLine();
}
}
}
Tuesday
template method design pattern c#
msmq set permissions c#
private void CreateQueue(string qname)
{
try
{
if (!MessageQueue.Exists(qname))
{
MessageQueue.Create(qname);
MessageQueue messageQueue = new MessageQueue(qname);
//messageQueue.SetPermissions("Everyone", MessageQueueAccessRights.FullControl);
messageQueue.SetPermissions("Local Service", MessageQueueAccessRights.FullControl);
}
}
catch (Exception e)
{
Debug.Write("Failed to setup queue:" + qname + ":" + e.ToString());
}
}
windows service installer class with event source creation
using System;
using System.Collections;
using System.Collections.Generic;
using System.ComponentModel;
using System.Configuration.Install;
using System.Diagnostics;
using System.Linq;
using System.ServiceProcess;
namespace ProcessingService
{
[RunInstaller(true)]
public partial class MyProcessorInstaller : System.Configuration.Install.Installer
{
private readonly ServiceProcessInstaller _processInstaller;
private readonly ServiceInstaller _svcInstaller;
public MyProcessorInstaller()
{
InitializeComponent();
_processInstaller = new ServiceProcessInstaller();
_svcInstaller = new ServiceInstaller();
_processInstaller.Account = ServiceAccount.LocalService;
_svcInstaller.StartType = ServiceStartMode.Automatic;
_svcInstaller.Description = "Describe here what service is doing. ";
_svcInstaller.DisplayName = "Processing Service";
_svcInstaller.ServiceName = "ProcessingService";
//Create Instance of EventLogInstaller
EventLogInstaller myEventLogInstaller = new EventLogInstaller();
// Set the Source of Event Log, to be created.
myEventLogInstaller.Source = "ProcessingService";
// Set the Log that source is created in
myEventLogInstaller.Log = "Application";
// Add myEventLogInstaller to the Installers Collection.
Installers.Add(myEventLogInstaller);
Installers.Add(_svcInstaller);
Installers.Add(_processInstaller);
}
}
}
reset sa password sql 2008
How to recover SA password on Microsoft SQL Server 2008 R2
When you are using MS SQL Server in mixed mode, it is very important that you know your SA password.
There can be different reasons you lost the password
- Person who installed the SQL Server knows the password but has left the building.
- You did not write down the password in your password file
- Password file is lost
- …
Steps to recover the SA password
-
Start SQL Server Configuration Manager
-
Stop the SQL services
-
Edit the properties of the SQL Service
-
Change the startup parameters of the SQL service by adding a –m; in front of the existing parameters
- Start the SQL services. These are now running in Single User Mode.
- Start CMD on tthe SQL server
-
Start the SQLCMD command. Now you will see following screen
-
Now we create a new user. Enter following commands
- CREATE LOGIN recovery WITH PASSWORD = ‘TopSecret 1′ (Remember SQL server has default strong password policy
-
Go
- Now this user is created
-
Now we grant the user a SYSADMIN roles using the same SQLCMD window.
- sp_addsrvrolemember ‘recovery’, ‘sysadmin’
- go
- Stop the SQL service again
-
Change the SQL service properties back to the default settings
- Start the SQL service again and use the new created login (recovery in my example)
-
Go via the security panel to the properties and change the password of the SA account.
- Now write down the new SA password.
Subscribe to:
Posts (Atom)
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
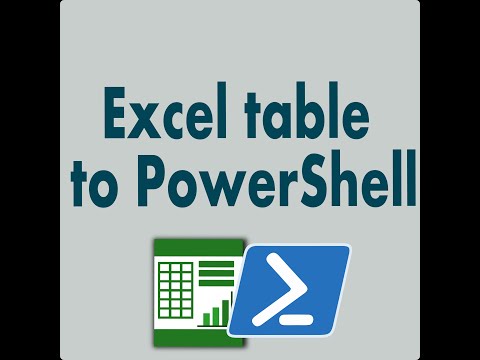
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
2010-11-24 Update: Please download latest version of Pidgin , that has this problem fixed , no additional steps required. Here are 3 ways to...