<script type="text/javascript">
$(document).ready(function () {
$("#SaveReportButton").click(function (event) {
event.preventDefault();
var $form = $(this).parents('form');
$.ajax({
type: "POST",
url: '@Url.Action("SaveDistributionReport")',
data: $form.serialize(),
error: function(xhr, status, error) {
//do something about the error
},
success: function(response) {
//save selected value
}
});
});
});
</script>
Friday
jquery ajax post form values
vim show tabstops
" highlight tabs and trailing spaces
set listchars=tab:>-,trail:-
set list
set tabstop=4
Monday
html.radiobuttonfor onclick for tracking change event
here is example how to use onclick event for tracking changes in radio button group.
so when radio button control will be changed , onPropertyChanged javascript function will be called passing value of radio button as argument.
@Html.LabelFor(m => m.BoolProperty)
@Html.RadioButtonFor(m => m.BoolProperty, true, new { onclick= "onPropertyChanged(true);" })
@StringResources.Submitted
@Html.RadioButtonFor(m => m.BoolProperty, false, new { onclick = "onPropertyChanged(false);" })
@StringResources.Fulfillment
so when radio button control will be changed , onPropertyChanged javascript function will be called passing value of radio button as argument.
Sunday
кожа для вордпресс, которую купили 27 тыс раз на сумму 1.5 мегабакса
кожа для вордпресс, которую купили 27 тыс раз на сумму 1.5 мегабакса
Вот тема для вордпресса, стоит $55. Ее продали 27+ тыс раз, на сумму 1.485 килобаксов (чуть менее 1.5 мегабакса). Самая продаваемая тема для вордпресса. Начало продаж 4 июня 2011.
Шароварщики нервно курят в сторонке (уж по соотношению доход/"затраты на разработку").
ЗЫ: попал на ту страничку увидев adwords на сайте sdelanounas.ru, сидя в инете с немецкого IP адреса. То есть магазин themeforest дает рекламу своих самых продаваемых продуктов.
Вот тема для вордпресса, стоит $55. Ее продали 27+ тыс раз, на сумму 1.485 килобаксов (чуть менее 1.5 мегабакса). Самая продаваемая тема для вордпресса. Начало продаж 4 июня 2011.
Шароварщики нервно курят в сторонке (уж по соотношению доход/"затраты на разработку").
ЗЫ: попал на ту страничку увидев adwords на сайте sdelanounas.ru, сидя в инете с немецкого IP адреса. То есть магазин themeforest дает рекламу своих самых продаваемых продуктов.
Friday
developer productivity metrics
- Productivity
- Does the developer get a reasonable amount of work done in a given period of time?
- Is the developer's velocity on bug fixing sufficient?
- Engagement
- Is the developer dedicated to his/her craft?
- Is the developer committed to delivering software on time?
- Is the developer dedicated to company success?
- Attention to Quality
- To what degree does the developer's code work as designed?
- Does the developer thoroughly test code and believe it to be correct before checking it in?
- Do a minimal number of bugs get reported against his/her code?
- Does the developer write unit tests for all new code?
- Does the developer follow the Boy Scout Rule and leave a module cleaner than it was before he or she worked on it?
- Code Base Knowledge and Management
- To what degree does the developer understand the code base assigned to him/her?
- Does the developer take responsibility for his/her team's code base, improving it at every opportunity?
- Adherence to coding guidelines and techniques
- Does developer's code routinely meet coding standards?
- Do code reviews reveal a minimum of problems and discrepancies?
- Does the developer use Dependency Injection to ensure decoupled code?
- Learning and Skills
- Is the developer constantly learning and improving his/her skills?
- Does the developer show a passion for the craft of software development?
- Personal Responsibility
- Does the developer first assume that the error lies within his or her code?
- Does the developer understand that he or she is solely responsible for their code working correctly?
- Does the developer take pride in their code, ensuring it is clean, tested, easy to read, and easy to maintain?
c# datetime beginning of day
This extension adds EndOfDay StartOfDay methods to DateTime so it's possible to call
DateFrom.Value.StartOfDay() or dateTo.Value.EndOfDay()
DateFrom.Value.StartOfDay() or dateTo.Value.EndOfDay()
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace AwesomeExtensions
{
public static class Extension
{
public static DateTime EndOfDay(this DateTime date)
{
return new DateTime(date.Year, date.Month, date.Day, 23, 59, 59, 999);
}
public static DateTime StartOfDay(this DateTime date)
{
return new DateTime(date.Year, date.Month, date.Day, 0, 0, 0, 0);
}
}
}
selenium popup example in c#
// waiting for popup to open
while (this.driver.WindowHandles.Count == 1)
{
this.WaitASec();
}
this.WaitASec();
// save the current window handle.
String parentWindowHandle = driver.CurrentWindowHandle;
IWebDriver popup = null;
var windowIterator = driver.WindowHandles;
bool onefound = false;
//enumerate through all windows to make sure at least one contains required text
foreach (var windowHandle in windowIterator)
{
popup = driver.SwitchTo().Window(windowHandle);
if (textPresent != "")
{
onefound=Regex.IsMatch(popup.FindElement(By.CssSelector("BODY")).Text, "^[\\s\\S]*" + textPresent + "[\\s\\S]*$");
}
}
Assert.IsTrue(onefound,"No text was found "+textPresent);
Wednesday
accessed from a thread other than the thread it was created on.
This happends when you are trying to modify control from another thread.You cannot do this directly it could be done only by invoker.
In order to resolve this situation please do following:
step 1: Create following extension
step2.Using developed extension:
Now in other thread instead of calling control directly like :
You have to call it as follows:
In order to resolve this situation please do following:
step 1: Create following extension
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows.Forms;
public static class Extension
{
public static void InvokeIfRequired(this Control control, MethodInvoker action)
{
if (control.InvokeRequired)
{
control.Invoke(action);
}
else
{
action();
}
}
}
step2.Using developed extension:
Now in other thread instead of calling control directly like :
htmlPanel1.Text = resml;
You have to call it as follows:
htmlPanel1.InvokeIfRequired(() =>{ htmlPanel1.Text = resml; });
Subscribe to:
Posts (Atom)
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
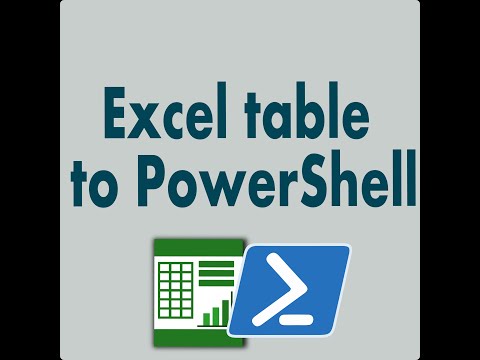
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
2010-11-24 Update: Please download latest version of Pidgin , that has this problem fixed , no additional steps required. Here are 3 ways to...