using System; using System.Runtime.Serialization; using System.Collections.Generic; using System.Text; using System.Text.RegularExpressions; /// <summary> /// Asserion block /// </summary> [Serializable] public class AssertionException : ApplicationException { public AssertionException(SerializationInfo info, StreamingContext context) : base(info, context) {} public AssertionException() : base() { } public AssertionException(string msg) : base(string.Format(msg)) { } /// <summary> /// Constructor /// </summary> /// <param name="msg"></param> /// <param name="obj"></param> public AssertionException(string msg, params object[] obj) :base(string.Format(msg, obj)) {} } public class Assertions { public void Throw(string message,params string[] objs ){ Throw(String.Format(message, objs)); } public void Throw(Exception exception) { throw exception; } public void Throw(string message) { var x = new AssertionException(message); throw (x); } public void IsInt(string num, string Message, out int result) { if (!int.TryParse(num,out result)) { Throw(Message); } } public void IsTrue(bool evaluation, string format, params string[] objects){ if (!evaluation){ Throw(format,objects ); } } public void IsFalse(bool evaluation, string format, params string[] objects){ if (evaluation) Throw(format, objects); } public void IsTrue(bool evaluation,string message){ if (!evaluation) Throw(message); } public void IsTrue(bool evaluation, Exception exception) { if (!evaluation) Throw(exception); } public void NotDBNull(Object evaluation, string message){ if (evaluation == DBNull.Value){ Throw(message); } } public void AllNotDBNull(string message,params object[] evaluation ) { foreach (object o in evaluation) { NotDBNull(o,message); } } public void AllNotDBNullOREmptyString(string message, params object[] evaluation) { foreach (object o in evaluation) { NotDBNull(o, message); NonEmptyString(o.ToString(), message); } } public void IsNotNull(Object evaluation,string message) { if (evaluation ==null) { Throw(message); } } public void AllNotNull( string message,params object[] evaluation) { foreach (object o in evaluation) { IsNotNull(o, message); } } public void AllNonEmptyString(string message, params object[] evaluation) { foreach (object o in evaluation) { IsNotNull(o,message); NonEmptyString(o.ToString(), message); } } public void NonEmptyString (string str,string message) { if (!(str!=null && str.Trim().Length >0)) { Throw(message); } } public void RegexMatch(string s, string reg,string message ) { Regex rg = new Regex(reg); IsTrue(rg.IsMatch(s), message); } public void RegexMatchAtLeastWithOne(string s, string[] reg, string message) { bool matched = false; foreach (string rega in reg) { Regex rg = new Regex(rega); if (rg.IsMatch(s)) { matched = true; break; } } IsTrue(matched, message); } }
Wednesday
assertive programming.assertion class
Below is assertion class.And here is programming example
Subscribe to:
Posts (Atom)
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
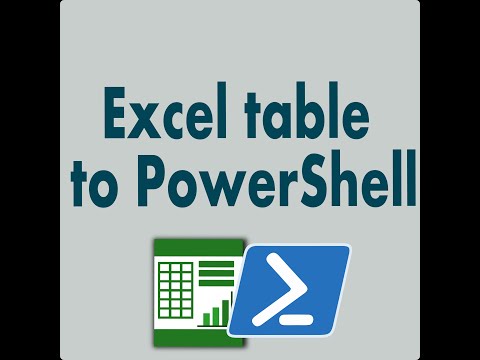
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
2010-11-24 Update: Please download latest version of Pidgin , that has this problem fixed , no additional steps required. Here are 3 ways to...