using System;
using System.Reflection;
using System.Text;
using Microsoft.Practices.EnterpriseLibrary.Caching;
using PostSharp.Aspects;
namespace Business.Shared.Attributes
{
[Serializable]
public sealed class CacheAttribute : OnMethodBoundaryAspect
{
// This field will be set by CompileTimeInitialize and serialized at build time,
// then deserialized at runtime.
private string _methodName;
// Method executed at build time.
public override void CompileTimeInitialize(MethodBase method, AspectInfo aspectInfo)
{
_methodName = method.DeclaringType.FullName + "." + method.Name;
}
private string GetCacheKey(object instance, Arguments arguments)
{
// If we have no argument, return just the method name so we don't uselessly allocate memory.
if (instance == null && arguments.Count == 0)
return _methodName;
// Add all arguments to the cache key. Note that generic arguments are not part of the cache
// key, so method calls that differ only by generic arguments will have conflicting cache keys.
var stringBuilder = new StringBuilder(_methodName);
stringBuilder.Append('(');
if (instance != null)
{
stringBuilder.Append(instance);
stringBuilder.Append("; ");
}
for (int i = 0; i < arguments.Count; i++)
{
stringBuilder.Append(arguments.GetArgument(i) ?? "null");
stringBuilder.Append(", ");
}
return stringBuilder.ToString();
}
// This method is executed before the execution of target methods of this aspect.
public override void OnEntry(MethodExecutionArgs args)
{
// Compute the cache key.
var cacheKey = GetCacheKey(args.Instance, args.Arguments);
// Fetch the value from the cache.
var cacheMgr = CacheFactory.GetCacheManager();
var value = cacheMgr.GetData(cacheKey);
if (value != null)
{
// The value was found in cache. Don't execute the method. Return immediately.
args.ReturnValue = value;
args.FlowBehavior = FlowBehavior.Return;
}
else
{
// The value was NOT found in cache. Continue with method execution, but store
// the cache key so that we don't have to compute it in OnSuccess.
args.MethodExecutionTag = cacheKey;
}
}
// This method is executed upon successful completion of target methods of this aspect.
public override void OnSuccess(MethodExecutionArgs args)
{
var cacheKey = (string)args.MethodExecutionTag;
var cacheMgr = CacheFactory.GetCacheManager();
cacheMgr.Add(cacheKey, args.ReturnValue);
}
}
}
Friday
Cache Attibute
Subscribe to:
Post Comments (Atom)
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
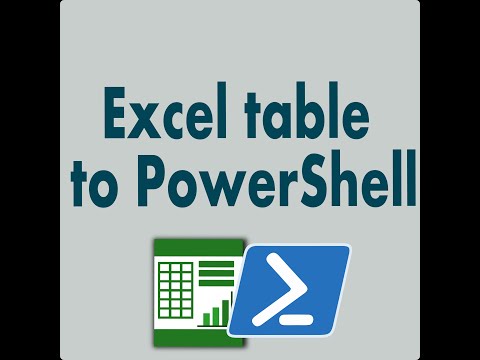
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
2010-11-24 Update: Please download latest version of Pidgin , that has this problem fixed , no additional steps required. Here are 3 ways to...
No comments:
Post a Comment