using System.Security;
public User Login(string login_name, string password){
ICredValidator cv = new LdapCredValidator(); //LanCredValidator();
User u = new User(conn);
if (cv.ValidateCred("MY-DOM", login_name, password))
{
// validation sucess
}else Throw("Login failed for {0}",login_name);
return u;
}
Friday
authentication in active directory
active directory - get computer lists
public void AD_ComputerList()
{
DirectoryEntry entry = new DirectoryEntry("LDAP://my-dom");
DirectorySearcher mySearcher = new DirectorySearcher(entry);
mySearcher.Filter = ("(objectClass=computer)");
Console.WriteLine("Listing of computers in the Active Directory");
Console.WriteLine("============================================");
foreach(SearchResult resEnt in mySearcher.FindAll())
{
Console.WriteLine("Name: {0}", resEnt.GetDirectoryEntry().Name.ToString());
}
Console.WriteLine("=========== End of Listing =============");
}
C# how to get current windows login
public void WinIdentity() {
System.Security.Principal.WindowsIdentity i = System.Security.Principal.WindowsIdentity.GetCurrent();
Console.WriteLine("name: {0}, token: {1}", i.Name, i.Groups[0].ToString());
Assert.IsNotNull(i);
}
webservice logging incoming/outgoing data
add following to web.config under system.web
<httpModules>
<add name="RequestResponseLogger" type="LoggingFilter, LoggingFilter"/>
</httpModules>
<customErrors mode="Off"/>
</system.web>
<microsoft.web.services2>
<diagnostics>
<trace enabled="true" input="D:\Logs\in.txt" output="D:\Logs\out.txt"/>
</diagnostics>
</microsoft.web.services2>
</configuration>
php function for dot.net webservice submission
<?
function web_service($serv,$plain_data){
$curl_handle = curl_init();
curl_setopt($curl_handle, CURLOPT_URL, "https://myserver.com/myService.asmx/".$serv);
curl_setopt($curl_handle, CURLOPT_HEADER, 1);
curl_setopt($curl_handle, CURLOPT_FOLLOWLOCATION, 1);
curl_setopt($curl_handle, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl_handle, CURLOPT_SSL_VERIFYPEER, 0);
curl_setopt($curl_handle, CURLOPT_SSL_VERIFYHOST, 0);
curl_setopt($curl_handle, CURLOPT_POST, 1);
curl_setopt($curl_handle, CURLOPT_POSTFIELDS, $plain_data);
$response = curl_exec ($curl_handle) or die ( "There has been an error connecting");
#echo $response;
curl_close ($curl_handle);
$response= strstr($response, '<?xml version="1.0" encoding="utf-8"?>');
$re = str_replace('>','>',str_replace('<','<',$response));
$re1= str_replace('<string xmlns="http://tempuri.org/">',"<string>",$re);
return $re1;
}
function web_method($f,$u){
$data="Subscriber=".$f['studid']."&Affiliate=".$u['referral']
$re1= msi_service("MyMethodName1",$data);
$xml = simplexml_load_string($re1);
$result = $xml->xpath("/string"); // <- parsing result
foreach($result as $result_code) {
return $result_code;
}
return $re1;
}
?>
here is post how to configure web service to allow remote post/get submission
rework dynamic sql into stored procedure with optional parameters
CREATE PROCEDURE TestProc
(
@Param1 varchar(50) = NULL,
@Param2 varchar(50) = NULL,
@Param3 varchar(50) = NULL
)
AS
SELECT
*
FROM
TestTable
WHERE
((@Param1 IS NULL) OR (col1 = @Param1)) AND
((@Param2 IS NULL) OR (col2 = @Param2)) AND
((@Param3 IS NULL) OR (col3 = @Param3))
Monday
c# property with using cache (expiration added)
public static int CacheDyration = 10;
/// <summary>
/// Cached Merchant table
/// </summary>
public static Hashtable MerchantHash
{
get
{
Hashtable retValue = null;
// Check if cache has Merchant saved
if (HttpContext.Current.Cache["MerchantHash"] != null)
{ // Return cached version
retValue = (Hashtable)HttpContext.Current.Cache["MerchantHash"];
}
else
{
// initializing new instance, you can change this initialization with using BLL classes
MerchantTableAdapter da = new MerchantTableAdapter();
retValue = Hashmaker.Hash(da.GetData(), "merchant_id", "merchantname");
if (retValue.Count > 0)
{
HttpContext.Current.Cache.Insert("MerchantHash", retValue, null, Cache.NoAbsoluteExpiration, new TimeSpan(0, CacheDyration, 0));
}
}
return retValue;
}
set
{ // Check if item is already in there
if (HttpContext.Current.Cache["MerchantHash"] != null)
{
// Remove old item
HttpContext.Current.Cache.Remove("MerchantHash");
} // Place a new one
HttpContext.Current.Cache.Insert("MerchantHash", value, null, Cache.NoAbsoluteExpiration, new TimeSpan(0, CacheDyration, 0));
}
}
clear cache in ASP.NET VB.NET
Private Sub ClearCache()
For Each CacheKey As DictionaryEntry In Cache
If CacheKey.Key.GetType.Equals(GetType(System.String)) Then
Dim MyKey As String = CacheKey.Key
Cache.Remove(CacheKey.Key)
End If
Next
End Sub
more...
Subscribe to:
Posts (Atom)
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
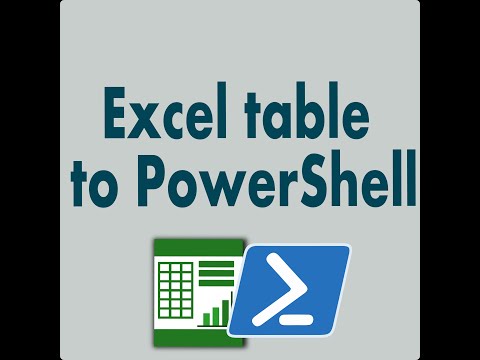
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
2010-11-24 Update: Please download latest version of Pidgin , that has this problem fixed , no additional steps required. Here are 3 ways to...