//step1.declare delagate public delegate T UnSafeProcedure<T>(); /*step2. add performance and error handling methods */ public string Concat(params object[] arguments) { StringBuilder sb = new StringBuilder(); foreach (var item in arguments) { sb.AppendFormat("\"{0}\",", item); } return sb.ToString(); } public T RunSafe<T>(UnSafeProcedure<T> s, params object[] arguments) { DateTime st = DateTime.Now; try { return s(); } catch (Exception e1) { { Debug.Write("Error," + Concat(arguments) + "\r\n" + e1.ToString()); } return default(T); } finally { var sec = DateTime.Now.Subtract(st).TotalSeconds; if (sec > 20) { string tm = ">20sec,start," + st.ToLongTimeString() + ",End," + DateTime.Now.ToLongTimeString(); Debug.Write(tm+ Concat(arguments)); } } } /*3.performance counting procedures will looks like:*/ public Receipt VerifyUser(int Id, string username, string password) { return RunSafe<Receipt>(delegate() { //internal procedure that needs to be chcked troubleshooted using (var service = Instance()) { return service.VerifyAccount(Id, username, password); } }, "VerifyUser,facilityId",Id,"username",username,"password",password); }
Monday
c# custom webservice logging and error handling
Subscribe to:
Post Comments (Atom)
here is powershell script on how to get list of files from changesets associated with one tfs task
$dllPath = "C:\Program Files (x86)\Microsoft Visual Studio\2019\Enterprise\Common7\IDE\CommonExtensions\Microsoft\TeamFoundation\...
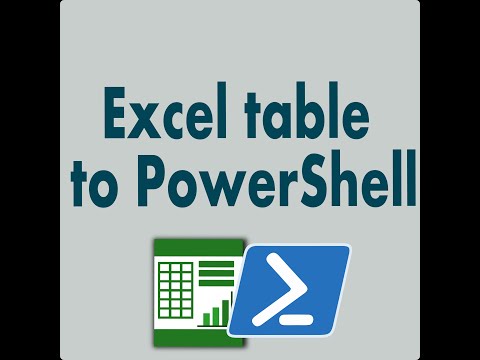
-
Error:The element 'Schedule' has invalid child element 'RecurrenceRule'. List of possible elements expected: 'Occurring...
-
$z = Import-Csv zerotrac.csv $nums = Import-Csv allleetcode.csv $md =@{} #converting one csv into hashmap for quicker search foreac...
-
Here is instruction how to make blinking text in rainmeter: 1.right click and select " Edit skin " 2.add following code to temp...
1 comment:
or parametreless function will be :
public void RunSafe(UnSafeVoid s, params object[] arguments)
{
DateTime st = DateTime.Now;
try
{
s();
}
catch (Exception e1)
{
{
log("Error," + Concat(arguments) + "\r\n" + e1.ToString());
}
}
finally
{
}
}
public delegate void UnSafeVoid();
Post a Comment